C语言,有一个一维数组,内放10个学生成绩,写一个函数,当主函数调用此函数后,能求出平均分、最高分和最低分。
时间: 2024-12-08 12:25:20 浏览: 29
在C语言中,你可以创建一个函数来计算一维数组的成绩总分、平均分、最高分和最低分。这里是一个简单的例子:
```c
#include <stdio.h>
// 定义结构体存储学生信息
typedef struct {
int score;
} Student;
// 函数原型声明
void calculate_scores(Student scores[], int size, float* avg, int* max_score, int* min_score);
int main() {
// 初始化学生分数数组
const int num_students = 10;
Student scores[num_students] = {95, 88, 92, 76, 85, 98, 78, 80, 90, 95};
// 分数指针初始化
float avg;
int max_score, min_score;
// 调用函数
calculate_scores(scores, num_students, &avg, &max_score, &min_score);
// 输出结果
printf("Average Score: %.2f\n", avg);
printf("Highest Score: %d\n", max_score);
printf("Lowest Score: %d\n", min_score);
return 0;
}
// 函数实现 - 计算并返回成绩信息
void calculate_scores(Student scores[], int size, float* avg, int* max_score, int* min_score) {
*avg = 0; // 初始化平均值
*max_score = scores[0]; // 假设第一个元素为初始最大值
*min_score = scores[0]; // 假设第一个元素为初始最小值
for (int i = 0; i < size; i++) {
*avg += scores[i]; // 累加平均值
if (scores[i] > *max_score) {
*max_score = scores[i];
}
if (scores[i] < *min_score) {
*min_score = scores[i];
}
}
*avg /= size; // 计算平均值
}
```
阅读全文
相关推荐
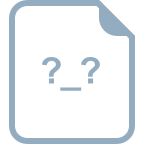















