用C++输入一行字符里,不要超过80个字符,含有数字和非数字字符以及空格等, 如果将其中所有连续出现的数字视为一个整数,要求统计在该字符串中共有多少个整数,并将这些数依次输出。
时间: 2024-12-17 18:20:38 浏览: 11
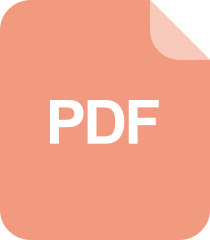
C++统计中英文大小写字母、数字、空格及其他字符个数的方法

在C++中,你可以通过遍历输入字符串、分割数字串并检查其是否为整数来实现这个功能。可以利用`std::isdigit()`函数判断字符是否为数字,同时维护一个计数器和一个临时字符串变量来存储连续的数字字符,直到遇到非数字字符为止。然后你可以尝试将这些数字转换成整数,如果转换成功说明是一个完整的整数,就增加计数并输出。以下是简单的步骤:
```cpp
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "请输入不超过80个字符的字符串: ";
getline(std::cin, input); // 防止换行符影响
int count = 0;
std::string num_str = ""; // 存储连续数字的字符串
for (char c : input) {
if (!std::isdigit(c)) { // 遇到非数字字符结束当前数字串
try {
int num = std::stoi(num_str); // 尝试将数字转换为整数
if (num != -1) { // 转换成功则计数并输出
std::cout << num << " ";
count++;
}
num_str.clear(); // 重置数字串
} catch (...) {
// 如果转换失败,清空数字串继续处理下一个字符
}
} else {
num_str += c; // 添加数字字符
}
}
// 检查最后一次循环中的完整数字
if (!num_str.empty()) {
try {
int num = std::stoi(num_str);
std::cout << num << " ";
count++;
} catch (...) {}
}
std::cout << "\n在字符串中有 " << count << " 个整数。\n";
return 0;
}
```
阅读全文
相关推荐
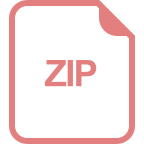
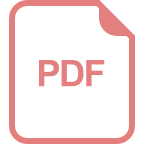















