java输入一组数进行选择排序
时间: 2024-10-02 12:04:04 浏览: 33
选择排序是一种简单直观的排序算法,它的工作原理是每一次从待排序的数据元素中选出最小(或最大)的一个元素,存放在序列的起始位置,直到全部待排序的数据元素排完。以下是使用Java编写的选择排序示例:
```java
import java.util.Scanner;
public class SelectionSort {
void selectionSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) { // 遍历数组
// 找到未排序部分的最小值索引
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
// 将找到的最小值与当前位置交换
if (minIndex != i) {
int temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入数字,以空格分隔:");
String input = scanner.nextLine();
int[] numbers = Arrays.stream(input.split("\\s+")).mapToInt(Integer::parseInt).toArray();
SelectionSort sorter = new SelectionSort();
sorter.selectionSort(numbers);
System.out.println("排序后的数组:");
for (int num : numbers) {
System.out.print(num + " ");
}
scanner.close();
}
}
```
在这个程序中,用户首先通过`Scanner`输入一系列整数,然后`selectionSort`方法会将它们按照升序排列。整个过程包括两层循环:外部循环用于控制遍历次数,内部循环用于寻找并替换当前位置的最小值。
阅读全文
相关推荐












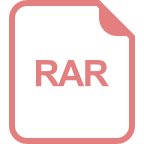


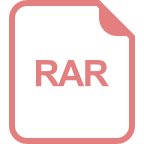

