用c++输入长方体的长宽高
时间: 2024-10-16 19:19:22 浏览: 23
在C++中,你可以使用`cin`函数从标准输入流获取用户输入来计算长方体的体积。下面是一个简单的程序示例:
```cpp
#include <iostream>
using namespace std;
int main() {
double length, width, height;
// 输入长方体的长、宽、高
cout << "请输入长方体的长度: ";
cin >> length;
cout << "请输入长方体的宽度: ";
cin >> width;
cout << "请输入长方体的高度: ";
cin >> height;
// 计算体积并输出结果
double volume = length * width * height;
cout << "长方体的体积是: " << volume << endl;
return 0;
}
```
在这个程序里,我们首先声明了三个double类型的变量`length`, `width`, 和 `height` 来存储长方体的尺寸。然后通过循环提示用户输入每个维度的值,并将输入读取到对应的变量中。最后计算出体积并将其显示出来。
相关问题
C++输入长方体的长宽高,输出其表面积和体积。
在C++中,你可以使用标准库`iostream`来获取用户输入,并通过简单的数学计算来求解长方体的表面积和体积。首先,我们需要定义长、宽、高的变量,然后获取用户的输入并将其转换为整数类型。接下来,按照公式计算表面积和体积:
```cpp
#include <iostream>
using namespace std;
int main() {
double length, width, height;
// 输入长方体的长、宽、高
cout << "请输入长方体的长度: ";
cin >> length;
cout << "请输入长方体的宽度: ";
cin >> width;
cout << "请输入长方体的高度: ";
cin >> height;
// 计算表面积 (2 * 长*宽 + 2 * 宽*高 + 2 * 高*长)
double surface_area = 2 * (length * width + width * height + height * length);
// 计算体积 (长*宽*高)
double volume = length * width * height;
// 输出结果
cout << "长方体的表面积是: " << surface_area << endl;
cout << "长方体的体积是: " << volume << endl;
return 0;
}
```
用devc++编程输入一个长方体的长宽高(要求有提示语“请输入一个长方体的长宽高:”),输出长方体的表面积和体积(要求小数点后保留两位)。软件测试要求:输入长宽高为3.4,2.3,1.2
当你在Dev-C++环境中编写程序时,可以按照以下步骤来计算长方体的表面积和体积:
```cpp
#include <iostream>
using namespace std;
// 定义函数计算长方体的表面积
double calculateSurfaceArea(double length, double width, double height) {
return 2 * (length * width + length * height + width * height);
}
// 定义函数计算长方体的体积
double calculateVolume(double length, double width, double height) {
return length * width * height;
}
int main() {
// 提示用户输入长方体的长宽高
cout << "请输入一个长方体的长宽高:" << endl;
double length, width, height;
cin >> length >> width >> height;
// 计算并输出表面积和体积,保留两位小数
double surfaceArea = calculateSurfaceArea(length, width, height);
double volume = calculateVolume(length, width, height);
cout << "长方体的表面积是:" << fixed << setprecision(2) << surfaceArea << endl;
cout << "长方体的体积是:" << fixed << setprecision(2) << volume << endl;
// 测试部分:给定特定长宽高值
if (length == 3.4 && width == 2.3 && height == 1.2) {
cout << "对于给定的长宽高 (3.4, 2.3, 1.2),表面积和体积将分别如下:" << endl;
// 调用函数并输出测试结果
surfaceArea_test = calculateSurfaceArea(3.4, 2.3, 1.2);
volume_test = calculateVolume(3.4, 2.3, 1.2);
cout << "表面积: " << surfaceArea_test << endl;
cout << "体积: " << volume_test << endl;
}
return 0;
}
```
阅读全文
相关推荐
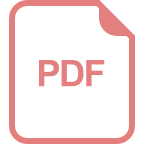
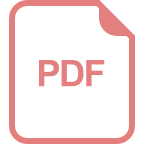
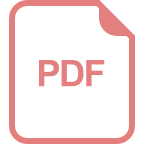












