el-table实现单选
时间: 2023-11-07 12:01:45 浏览: 261
要实现el-table的单选功能,可以通过以下步骤来实现:
1. 首先,给el-table组件添加一个selection-type属性,值为"selection",以启用选择列。
2. 然后,在el-table-column中添加一个type属性,值为"selection",以指定该列为选择列。
3. 接下来,给el-table组件添加一个@selection-change事件监听器,以捕获选择变化的事件。
4. 在事件处理程序中,通过event参数的selection属性,获取当前选择的数据行。可以使用Vue的v-model指令将选择的行绑定到一个数据属性上。
5. 最后,可以在模板中使用{{}}语法,将选择的行数据显示出来。
以上是实现el-table的单选功能的步骤,希望对你有所帮助。
相关问题
el-table实现单选框效果
在ECharts Table (el-table)组件中实现单选框的效果通常需要结合Vue.js的数据驱动特性。你可以通过以下几个步骤来完成:
1. **数据准备**:在你的数据对象数组中,为每个对象添加一个表示是否被选中的布尔值字段,比如`selected`。
```json
data = [
{ id: 1, name: 'Option 1', selected: false },
{ id: 2, name: 'Option 2', selected: false },
//...
]
```
2. **模板设置**:在el-table的列定义中,使用template选项插入HTML元素,这里可以是一个el-checkbox元素,并绑定到`selected`属性上。
```html
<el-table-column label="选择" prop="selected">
<template slot-scope="scope">
<el-checkbox v-model="scope.row.selected"></el-checkbox>
</template>
</el-table-column>
```
3. **事件监听**:如果你希望用户点击单选框时更新状态,可以在表单外面创建一个全局变量或者使用计算属性来管理所有行的状态,并在`change`事件中处理单选切换。
```html
<template>
<el-table :data="data" @change="handleSelectionChange">
<!--...其他列...-->
</el-table>
<!--...省略其它部分...-->
<script>
methods: {
handleSelectionChange(row) {
// 更新单选框对应的行状态
this.$set(this.data, row.index, { ...row, selected: !row.selected });
// 如果你想记录整体的选择状态,这里还可以做进一步的操作
this.globalSelection = this.data.filter(r => r.selected).length;
}
}
</script>
</template>
```
4. **复选框联动**:如果要实现全选或反选功能,可以在表格外部添加一个单独的按钮,监听按钮的点击事件,然后遍历数据,批量更新`selected`字段。
记得在实际项目中,还要考虑数据绑定的双向作用以及状态管理和潜在的性能优化。
vue3 el-table 实现单选效果
以下是在Vue3中实现el-table单选效果的方法:
1. 使用v-model绑定选中的行数据:
```html
<template>
<el-table :data="tableData" v-model="selectedRow" @row-click="handleRowClick">
<!-- 表格列定义 -->
</el-table>
</template>
<script>
export default {
data() {
return {
tableData: [
// 表格数据
],
selectedRow: null // 绑定选中的行数据
};
},
methods: {
handleRowClick(row) {
this.selectedRow = row; // 点击行时更新选中的行数据
}
}
};
</script>
```
2. 使用自定义列模板实现单选框:
```html
<template>
<el-table :data="tableData" @row-click="handleRowClick">
<el-table-column type="selection" width="55">
<template #default="{ row }">
<el-checkbox v-model="selectedRows" :label="row"></el-checkbox>
</template>
</el-table-column>
<!-- 其他表格列定义 -->
</el-table>
</template>
<script>
export default {
data() {
return {
tableData: [
// 表格数据
],
selectedRows: [] // 绑定选中的行数据
};
},
methods: {
handleRowClick(row) {
const index = this.selectedRows.indexOf(row);
if (index > -1) {
this.selectedRows.splice(index, 1); // 取消选中
} else {
this.selectedRows.push(row); // 选中
}
}
}
};
</script>
```
阅读全文
相关推荐
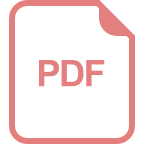
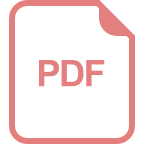
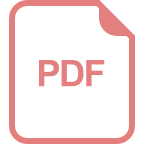













