如何指定mode,举个例子
时间: 2024-09-22 13:05:08 浏览: 25
在pandas的`to_excel`函数中,你可以通过`mode`参数来控制写入Excel文件的行为。`mode`可以有以下几个选项:
- `'w'`(默认值):完全写入模式,会清除文件中原有的所有内容,并创建一个新的工作簿。
- `'a'`:追加模式,如果文件已存在,将在其末尾追加新的数据;如果文件不存在,它会被创建。
- `'x'`:只写模式,如果文件存在,则抛出错误;如果文件不存在,它会被创建。
- `'b'`:二进制模式,通常用于写入包含图片、公式等二进制数据的文件。
例如,如果你想在已存在的文件“test.xlsx”中追加数据,你可以这样做:
```python
import pandas as pd
# 假设df是要写入的数据
df_to_write = pd.DataFrame({'Name': ['Alice', 'Bob'], 'Age': [25, 30]})
# 指定mode为'a'追加
df_to_write.to_excel('test.xlsx', mode='a', index=False)
```
在这个例子中,如果“test.xlsx”已经存在,`to_excel`会将其追加到现有数据的底部。如果没有该文件,它将会创建一个新的Excel文件。
相关问题
GPIO_MODE_IPU stm32举个例子
GPIO_MODE_IPU是STM32系列微控制器中的一种GPIO配置模式,它代表Input Protection Output(输入保护输出)。这种模式下,GPIO口可以作为输入端口,同时提供过电压和上拉电流保护功能,防止外部信号对内部电路造成损害。
例如,在STM32F103中,如果你有一个GPIOB端口想要设置为IPU模式,你可以这样做:
```c
// 导入头文件
#include "stm32f10x_gpio.h"
void configure_GPIO_B_for_IPU(void) {
// 定义GPIOB的起始地址
GPIO_TypeDef *GPIOB = GPIOA;
// 设置GPIOB的某一个引脚(如PB5)为输入保护输出
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_PIN_5; // PB5
GPIO_InitStruct.GPIO_Mode = GPIO_MODE_IPU;
GPIO_InitStruct.GPIO_Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.GPIO_PuPd = GPIO_NOPULL; // 关闭上拉
HAL_GPIO_Init(GPIOB, &GPIO_InitStruct);
}
```
在这个例子中,`GPIO_InitStruct.GPIO_Mode = GPIO_MODE_IPU` 表示将PB5设置为输入保护模式。然后你可以通过`HAL_GPIO_ReadPin(GPIOB, GPIO_PIN_5)`读取该引脚的状态,而不用担心外部信号的影响。
写个c程序举个例子
好的,以下是一个简单的 C 语言程序,用于获取 DRM 设备的当前帧缓冲对象并将其保存为 BMP 图像文件:
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/fb.h>
#include <linux/drm.h>
#include <drm/drm_fourcc.h>
#define WIDTH 800
#define HEIGHT 600
int main(int argc, char **argv)
{
// 打开 DRM 设备
int fd = open("/dev/dri/card0", O_RDWR);
if (fd < 0) {
fprintf(stderr, "failed to open DRM device\n");
exit(EXIT_FAILURE);
}
// 获取当前显示的帧缓冲对象
drmModeRes *resources = drmModeGetResources(fd);
if (!resources) {
fprintf(stderr, "failed to get DRM resources\n");
close(fd);
exit(EXIT_FAILURE);
}
drmModeConnector *connector = NULL;
drmModeEncoder *encoder = NULL;
drmModeCrtc *crtc = NULL;
for (int i = 0; i < resources->count_connectors; i++) {
connector = drmModeGetConnector(fd, resources->connectors[i]);
if (connector->connection == DRM_MODE_CONNECTED) {
for (int j = 0; j < connector->count_encoders; j++) {
encoder = drmModeGetEncoder(fd, connector->encoders[j]);
if (encoder->crtc_id) {
crtc = drmModeGetCrtc(fd, encoder->crtc_id);
break;
}
drmModeFreeEncoder(encoder);
}
}
drmModeFreeConnector(connector);
if (crtc) break;
}
if (!crtc) {
fprintf(stderr, "failed to get current CRTC\n");
drmModeFreeResources(resources);
close(fd);
exit(EXIT_FAILURE);
}
// 获取当前帧缓冲对象的信息
drmModeFB *fb = drmModeGetFB(fd, crtc->buffer_id);
if (!fb) {
fprintf(stderr, "failed to get current FB\n");
drmModeFreeCrtc(crtc);
drmModeFreeResources(resources);
close(fd);
exit(EXIT_FAILURE);
}
if (fb->width != WIDTH || fb->height != HEIGHT) {
fprintf(stderr, "current FB size is not %dx%d\n", WIDTH, HEIGHT);
drmModeFreeFB(fb);
drmModeFreeCrtc(crtc);
drmModeFreeResources(resources);
close(fd);
exit(EXIT_FAILURE);
}
// 映射帧缓冲对象到用户空间
uint32_t *buf = NULL;
struct drm_mode_map_dumb map_dumb = {
.handle = fb->handle,
};
if (ioctl(fd, DRM_IOCTL_MODE_MAP_DUMB, &map_dumb) < 0) {
fprintf(stderr, "failed to map dumb buffer\n");
drmModeFreeFB(fb);
drmModeFreeCrtc(crtc);
drmModeFreeResources(resources);
close(fd);
exit(EXIT_FAILURE);
}
buf = mmap(NULL, fb->size, PROT_READ, MAP_SHARED, fd, map_dumb.offset);
if (buf == MAP_FAILED) {
fprintf(stderr, "failed to mmap dumb buffer\n");
drmModeFreeFB(fb);
drmModeFreeCrtc(crtc);
drmModeFreeResources(resources);
close(fd);
exit(EXIT_FAILURE);
}
// 转换像素格式为 RGB565
uint16_t *rgb_buf = (uint16_t *)malloc(WIDTH * HEIGHT * sizeof(uint16_t));
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
uint32_t pixel = buf[i * fb->pitch / 4 + j];
uint16_t r = (pixel >> 16) & 0xff;
uint16_t g = (pixel >> 8) & 0xff;
uint16_t b = pixel & 0xff;
rgb_buf[i * WIDTH + j] = ((r >> 3) << 11) | ((g >> 2) << 5) | (b >> 3);
}
}
// 保存为 BMP 图像文件
FILE *fp = fopen("drm_fb.bmp", "wb");
if (fp) {
uint8_t bmp_header[54] = {
0x42, 0x4d, 0x36, 0x20, 0x0c, 0x00, 0x00, 0x00, 0x00, 0x00,
0x36, 0x00, 0x00, 0x00, 0x28, 0x00, 0x00, 0x00, 0x20, 0x03,
0x00, 0x00, 0xe0, 0x01, 0x00, 0x00, 0x01, 0x00, 0x10, 0x00,
0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x20, 0x0c, 0x00,
0x00, 0x13, 0x0b, 0x00, 0x00, 0x13, 0x0b, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00,
};
uint32_t row_size = WIDTH * 2;
uint32_t file_size = sizeof(bmp_header) + row_size * HEIGHT;
uint32_t padding_size = (4 - (row_size % 4)) % 4;
uint8_t padding[4] = {0};
bmp_header[2] = file_size;
bmp_header[6] = file_size >> 8;
bmp_header[10] = sizeof(bmp_header);
bmp_header[18] = WIDTH;
bmp_header[19] = WIDTH >> 8;
bmp_header[20] = WIDTH >> 16;
bmp_header[21] = WIDTH >> 24;
bmp_header[22] = HEIGHT;
bmp_header[23] = HEIGHT >> 8;
bmp_header[24] = HEIGHT >> 16;
bmp_header[25] = HEIGHT >> 24;
fwrite(bmp_header, 1, sizeof(bmp_header), fp);
for (int i = 0; i < HEIGHT; i++) {
fwrite(rgb_buf + (HEIGHT - i - 1) * WIDTH, 1, row_size, fp);
fwrite(padding, 1, padding_size, fp);
}
fclose(fp);
}
// 释放资源
free(rgb_buf);
munmap(buf, fb->size);
drmModeFreeFB(fb);
drmModeFreeCrtc(crtc);
drmModeFreeResources(resources);
close(fd);
return 0;
}
```
注意,该程序只是一个简单的示例,实际应用中还需要考虑各种异常情况的处理。
阅读全文
相关推荐
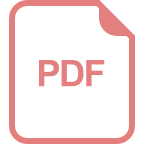
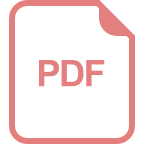
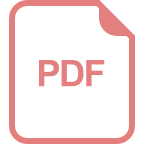













