用c语言:输入一行字符,分别统计出其中英文字母、空格、数字和其他字符的个数。
时间: 2023-11-23 20:56:49 浏览: 180
以下是用C语言统计一行字符中英文字母、空格、数字和其他字符个数的代码:
```c
#include <stdio.h>
int main() {
char c;
int ch, s, d, o;
ch = 0;
s = 0;
d = 0;
o = 0;
while ((c = getchar()) != '\n') {
if (c >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z')
ch++;
else if (c == ' ')
s++;
else if (c >= '0' && c <= '9')
d++;
else
o++;
}
printf("char=%d space=%d digit=%d others=%d\n", ch, s, d, o);
return 0;
}
```
相关问题
C语言:输入一行字符,分别统计出其中英文字母、空格、数字和其他字符的个数
好的,这个问题属于技术问题。以下是解答:
您可以使用一个循环来遍历输入的字符串,然后使用isdigit()、isalpha()、isspace()等函数来判断字符是否属于英文字母、数字或空格。然后分别统计它们的个数即可。代码示例:
```
#include<stdio.h>
#include<ctype.h>
int main(){
int alphabets=0, digits=0, spaces=0, others=0;
char input[100];
printf("请输入一行字符:");
fgets(input, 100, stdin);
for(int i=0; input[i]!='\0'; i++){
if(isalpha(input[i])){
alphabets++;
}
else if(isdigit(input[i])){
digits++;
}
else if(isspace(input[i])){
spaces++;
}
else{
others++;
}
}
printf("英文字母个数:%d\n", alphabets);
printf("数字个数:%d\n", digits);
printf("空格个数:%d\n", spaces);
printf("其他字符个数:%d\n", others);
return 0;
}
```
您可以将输入的字符串存储在字符数组input中,并使用fgets()函数从标准输入中读取它。然后,循环遍历输入字符串中的每个字符,并使用相应的函数判断字符属于哪一类。然后,增加相应计数器的值。最后,输出四个计数器的值。
用c语言:输入一行字符,分别统计出其中英文字母、空格、数字和其他字符的个数。
以下是用C语言统计一行字符中英文字母、空格、数字和其他字符个数的代码:
```c
#include <stdio.h>
int main() {
char c;
int ch, s, d, o;
ch = 0;
s = 0;
d = 0;
o = 0;
while ((c = getchar()) != '\n') {
if (c >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z')
ch++;
else if (c == ' ')
s++;
else if (c >= '0' && c <= '9')
d++;
else
o++;
}
printf("char=%d space=%d digit=%d others=%d\n", ch, s, d, o);
return 0;
}
```
相关推荐
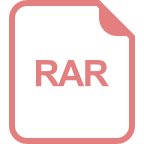
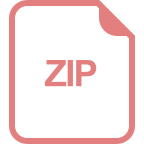












