那就麻烦你给我提供一个AES的java工具类, 并且使用ECB加密,zeroPadding填充, 数据块为128位, 密码为 1234567890abcdef
时间: 2024-04-21 13:25:03 浏览: 53
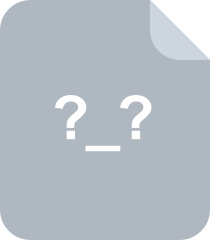
AESUtils实现了基于AES的ECB模式,选用了zeropadding填充,数据位为128 加上密码去加解密数据(优化并实测通过)
以下是一个使用AES/ECB/ZeroPadding加密的Java工具类示例:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class AESUtil {
private static final String ALGORITHM = "AES";
private static final String TRANSFORMATION = "AES/ECB/ZeroPadding";
public static String encrypt(String plainText, String password) throws Exception {
SecretKeySpec secretKeySpec = generateKey(password);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
byte[] encryptedBytes = cipher.doFinal(padString(plainText).getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String encryptedText, String password) throws Exception {
SecretKeySpec secretKeySpec = generateKey(password);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedText));
return removePadding(new String(decryptedBytes, StandardCharsets.UTF_8));
}
private static SecretKeySpec generateKey(String password) throws Exception {
byte[] keyBytes = password.getBytes(StandardCharsets.UTF_8);
return new SecretKeySpec(keyBytes, ALGORITHM);
}
private static String padString(String input) {
int paddingLength = 16 - (input.length() % 16);
StringBuilder paddedInput = new StringBuilder(input);
for (int i = 0; i < paddingLength; i++) {
paddedInput.append('\0');
}
return paddedInput.toString();
}
private static String removePadding(String input) {
return input.replaceAll("\0+$", "");
}
public static void main(String[] args) {
try {
String plainText = "Hello, World!";
String password = "1234567890abcdef";
String encryptedText = encrypt(plainText, password);
System.out.println("Encrypted Text: " + encryptedText);
String decryptedText = decrypt(encryptedText, password);
System.out.println("Decrypted Text: " + decryptedText);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
上述代码使用了AES/ECB/ZeroPadding加密算法,并提供了`encrypt`和`decrypt`方法用于加密和解密操作。在示例中,明文为"Hello, World!",密码为"1234567890abcdef"。运行代码后,会输出加密后的密文和解密后的明文。请注意,使用ECB模式并且只使用ZeroPadding填充可能会存在安全性问题,建议使用更加安全的加密模式和填充方式。
阅读全文
相关推荐
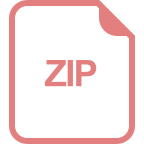
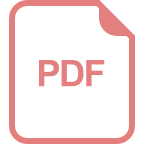
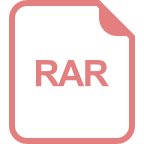
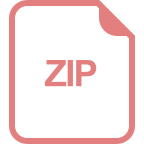
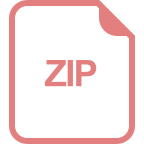
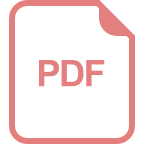
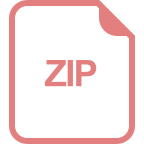
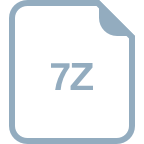
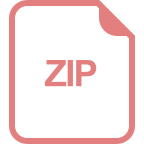
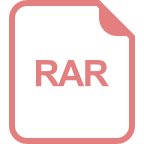
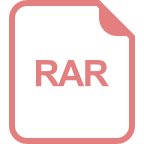
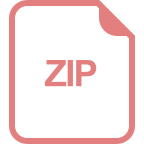
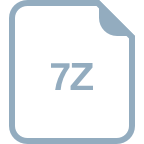
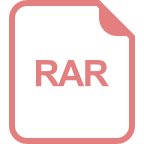
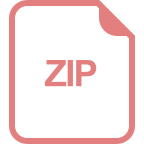
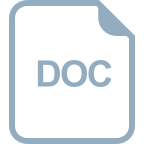