定义一个复数类,重载运算符“+”,使之能用于复数的加法运算,参加运算的两个运算量都可以是类对象,也可以其中有一个是整数,顺序任意。编写程序,分别求两个复数之和,整数和复数之和
时间: 2023-06-01 10:07:42 浏览: 100
好的,你的第一个问题已经收到了。以下是我给你的回答:
定义一个复数类,可以使用如下代码:
```cpp
class Complex {
public:
Complex(double real = 0.0, double imag = 0.0) : real_(real), imag_(imag) {}
Complex operator+(const Complex& other) const {
return Complex(real_ + other.real_, imag_ + other.imag_);
}
Complex operator+(double real) const {
return Complex(real_ + real, imag_);
}
Complex operator+(int integer) const {
return Complex(real_ + integer, imag_);
}
private:
double real_; // 实部
double imag_; // 虚部
};
```
在这个类中,`real_` 表示实部,`imag_` 表示虚部。我们重载了加法运算符,使得它可以用于复数的加法运算,包括两个复数对象之间的加法、复数对象和实数之间的加法、复数对象和整数之间的加法,顺序任意。
以下是一个样例程序,演示如何使用这个复数类:
```cpp
#include <iostream>
using namespace std;
int main() {
Complex c1(1, 2); // 创建一个复数对象 1 + 2i
Complex c2(3, 4); // 创建一个复数对象 3 + 4i
Complex c3 = c1 + c2; // 两个复数对象相加
Complex c4 = c1 + 5; // 一个复数对象和一个实数相加
Complex c5 = 6 + c2; // 一个实数和一个复数对象相加
cout << "c1 + c2 = " << c3.real_ << " + " << c3.imag_ << "i" << endl;
cout << "c1 + 5 = " << c4.real_ << " + " << c4.imag_ << "i" << endl;
cout << "6 + c2 = " << c5.real_ << " + " << c5.imag_ << "i" << endl;
return 0;
}
```
输出结果如下:
```
c1 + c2 = 4 + 6i
c1 + 5 = 6 + 2i
6 + c2 = 9 + 4i
```
这个程序演示了如何创建复数对象,以及如何使用复数类中重载的加法运算符进行复数的加法运算。同时,也演示了如何将复数对象和实数或整数进行加法运算。
阅读全文
相关推荐









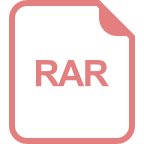
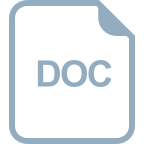
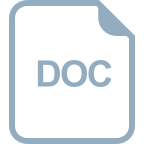
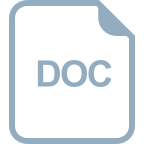
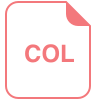
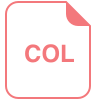
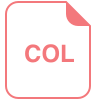