编写一个程序,接受用户输入的一行英文句子(假设该句子仅由英文单词及空格构成,不包括逗号等符号),统计并输出该行句子包含的单词个数及单词的平均长度。
时间: 2024-05-16 09:15:55 浏览: 126
可以利用 Python 的字符串和列表操作来完成这个任务。
以下是一个简单的实现:
```python
sentence = input("请输入一行英文句子:")
# 统计单词个数
word_count = len(sentence.split())
# 计算平均单词长度
words = sentence.split()
total_len = sum(len(word) for word in words)
avg_len = total_len / word_count
print("单词个数:", word_count)
print("平均单词长度:", avg_len)
```
首先,我们使用 `input` 函数从用户那里获取输入的句子。然后,我们使用字符串的 `split` 方法将句子分割成单词,这将返回一个包含单词的列表。我们使用 `len` 函数计算单词的数量。
接下来,我们使用一个列表推导式计算所有单词的长度总和。然后,我们将总长度除以单词数以得到平均长度。
最后,我们使用 `print` 函数输出结果。
相关问题
编写一个程序接受用户输入的一行英文句子(假设该句子仅由英文单词及空格构成,不包括逗号等符号),统计并输出该行句子包含的单词个数及单词的平均长度
好的,这个问题我可以回答。
请看以下代码:
```
#include <iostream>
#include <string>
using namespace std;
int main() {
string sentence;
getline(cin, sentence); // 获取用户输入的一行英文句子
int count = 0;
double total_length = 0.0;
for (int i = 0; i < sentence.length(); i++) {
if (sentence[i] == ' ') { // 判断是不是空格
count++; // 统计单词个数
}
else {
total_length++; // 统计单词长度
}
}
count++; // 最后一个单词没有空格,要手动加一个
double average_length = total_length / count; // 计算平均长度
cout << "单词个数:" << count << endl;
cout << "平均长度:" << average_length << endl;
return 0;
}
```
该程序先用 `getline()` 方法获得用户输入的一行英文句子。然后用 `for` 循环遍历每个字符,如果是空格就统计单词个数,否则就累加单词长度。最后手动加上最后一个单词,并计算平均长度,最终输出结果。
希望我的回答能够帮到您!
编写一个程序 接受用户输入一行英文句子(假设该句子仅由英文单词及空格构成,不包括逗号等符号),统计并输出该句子包含的单词个数及单词的平均长度。
编写这样一个程序可以分为以下几个步骤:
1. **获取用户输入**:首先,你需要创建一个函数来接收用户的输入,例如在Python中可以使用`input()`函数。
```python
def get_user_input():
sentence = input("请输入一行英文句子:")
return sentence.strip()
```
2. **分割单词**:然后,我们需要将句子按空格分割成单词列表。Python的`split()`函数非常适合这个任务。
```python
def split_words(sentence):
words = sentence.split(' ')
return words
```
3. **计算单词个数**:遍历列表,计算单词的数量。
```python
def count_words(words):
word_count = len(words)
return word_count
```
4. **计算平均单词长度**:对于每个单词,获取其长度并累加,最后除以单词总数得到平均长度。
```python
def average_word_length(words):
total_length = sum(len(word) for word in words)
if word_count > 0:
average_length = total_length / word_count
return average_length
else:
return 0 # 防止除零错误,当没有单词时返回0
```
5. **整合所有功能**:将上述部分组合起来,并打印结果。
```python
def main():
sentence = get_user_input()
words = split_words(sentence)
word_count = count_words(words)
avg_length = average_word_length(words)
print(f"句子中有 {word_count} 个单词,平均单词长度为 {avg_length:.2f} 字符.")
if __name__ == "__main__":
main()
```
阅读全文
相关推荐
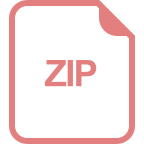
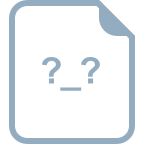




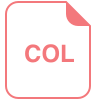
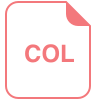
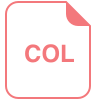
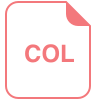
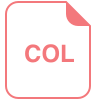
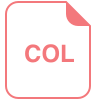
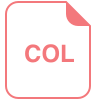
