node mongoose 多表关联删除
时间: 2023-07-07 07:21:10 浏览: 63
在 Node.js 中使用 Mongoose 进行多表关联删除,需要使用到 Mongoose 的 middleware 中的 pre 钩子函数进行操作。假设我们有两个模型: User 和 Post,User 模型中有一个数组类型的属性 posts,存储该用户发布的所有文章的 _id。我们需要在删除某篇文章时,同时将该文章的 _id 从所有用户的 posts 数组中删除。
代码实现如下:
```javascript
const mongoose = require('mongoose');
const UserSchema = new mongoose.Schema({
name: {
type: String,
required: true
},
posts: [{
type: mongoose.Schema.Types.ObjectId,
ref: 'Post'
}]
});
const PostSchema = new mongoose.Schema({
title: {
type: String,
required: true
},
content: {
type: String,
required: true
}
});
// 在 Post 模型中定义删除文章前的操作
PostSchema.pre('remove', function(next) {
const post = this;
// 从 User 模型中删除该文章的 _id
mongoose.model('User').updateMany({ posts: post._id }, { $pull: { posts: post._id } }, next);
});
const User = mongoose.model('User', UserSchema);
const Post = mongoose.model('Post', PostSchema);
// 删除一篇文章
Post.findOne({ title: '要删除的文章标题' }, function(err, post) {
if (err) {
console.log(err);
} else {
post.remove();
}
});
```
在 Post 模型中,我们使用 pre('remove', function(next) {}) 定义了删除文章前的操作。在该操作中,我们首先获取即将删除的文章 post,然后通过 mongoose.model('User').updateMany() 删除 User 模型中所有 posts 数组中包含该文章 _id 的元素。其中,$pull 操作符表示从数组中删除指定元素。
需要注意的是,我们在 User 模型的 posts 属性中使用了 ref 关联到了 Post 模型,这样才能在删除文章时找到所有包含该文章 _id 的 User,从而进行删除操作。
相关推荐
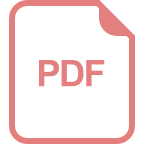
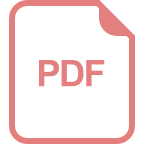
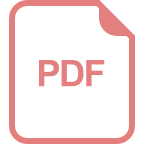














