python 数据库编程 实现学生管理中数据的导入及显示
时间: 2024-04-01 20:31:56 浏览: 48
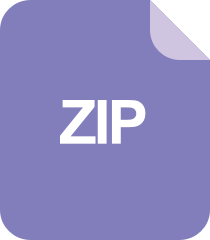
数据库大作业-基于python实现学生信息管理系统+源代码+文档说明+er图+结构图
首先,需要安装Python的数据库模块,例如MySQLdb、pymysql或sqlite3。
然后,可以通过以下步骤实现学生管理中数据的导入及显示:
1. 连接数据库:
```python
import pymysql
db = pymysql.connect(host='localhost', user='root', password='password', db='test')
cursor = db.cursor()
```
2. 创建学生表:
```python
sql = """
CREATE TABLE students (
id INT(10) PRIMARY KEY,
name VARCHAR(20),
age INT(3),
gender VARCHAR(10),
grade VARCHAR(20)
)
"""
cursor.execute(sql)
```
3. 导入学生数据:
```python
data = [
(1, 'Tom', 18, 'Male', 'Grade 1'),
(2, 'Jerry', 19, 'Male', 'Grade 2'),
(3, 'Lucy', 17, 'Female', 'Grade 1'),
(4, 'Lily', 18, 'Female', 'Grade 3')
]
for d in data:
sql = "INSERT INTO students(id, name, age, gender, grade) VALUES (%s, %s, %s, %s, %s)"
cursor.execute(sql, d)
db.commit()
```
4. 查询学生数据并显示:
```python
sql = "SELECT * FROM students"
cursor.execute(sql)
result = cursor.fetchall()
for r in result:
print(r)
```
完整代码示例:
```python
import pymysql
db = pymysql.connect(host='localhost', user='root', password='password', db='test')
cursor = db.cursor()
sql = """
CREATE TABLE students (
id INT(10) PRIMARY KEY,
name VARCHAR(20),
age INT(3),
gender VARCHAR(10),
grade VARCHAR(20)
)
"""
cursor.execute(sql)
data = [
(1, 'Tom', 18, 'Male', 'Grade 1'),
(2, 'Jerry', 19, 'Male', 'Grade 2'),
(3, 'Lucy', 17, 'Female', 'Grade 1'),
(4, 'Lily', 18, 'Female', 'Grade 3')
]
for d in data:
sql = "INSERT INTO students(id, name, age, gender, grade) VALUES (%s, %s, %s, %s, %s)"
cursor.execute(sql, d)
db.commit()
sql = "SELECT * FROM students"
cursor.execute(sql)
result = cursor.fetchall()
for r in result:
print(r)
db.close()
```
以上代码演示了如何使用Python进行数据库编程,实现学生管理中数据的导入及显示。
阅读全文
相关推荐
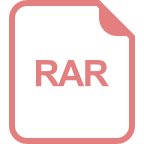
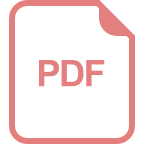
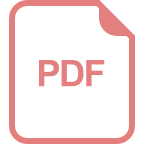
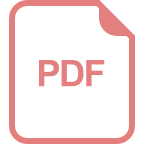
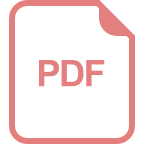
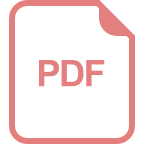
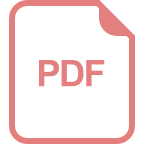
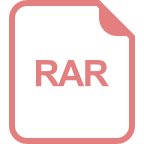
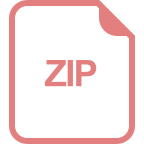
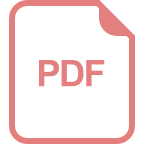
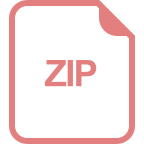
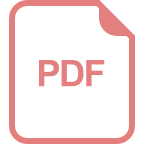
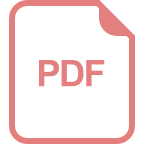
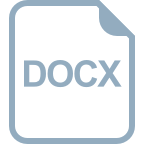
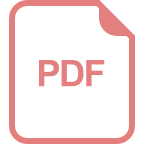