Write the following code in c #,7. Write a method with the following specifications: name: DisplayEquation arguments: none return value: nothing displays: the following table: x 5 3x -2x^2 y 0.0 5.0 0.0 0.0 5.0 0.3 5.0 0.8 -0.1 5.6 0.5 5.0 1.5 -0.5 6.0 0.8 5.0 2.3 -1.1 6.1 1.0 5.0 3.0 -2.0 6.0 1.3 5.0 3.8 -3.1 5.6 1.5 5.0 4.5 -4.5 5.0 1.8 5.0 5.3 -6.1 4.1 2.0 5.0 6.0 -8.0 3.0 This table is calculated using the quadratic equation y = 5 + 3x -2x2 The value of x starts at 0 and moves in increments of 0.25 to 2.0. (The first column is misleading because the last digit is rounded up i.e. 0.25 is displayed as 0.3and. 0.75 is displayed as 0.8) Modify your DisplayMenu method by adding another choice below the last item. In your main when the user enters the appropriate choice in response to the menu choices, you will invoke this method and to display the above values
时间: 2024-01-30 15:04:24 浏览: 87
Sure, here's the C# code that meets the specifications you provided:
```
using System;
public class Program {
public static void Main(string[] args) {
DisplayMenu();
int choice = int.Parse(Console.ReadLine());
switch (choice) {
case 1:
// do something for choice 1
break;
case 2:
// do something for choice 2
break;
case 3:
// do something for choice 3
break;
case 4:
DisplayEquation();
break;
default:
Console.WriteLine("Invalid choice. Please choose again.");
break;
}
}
public static void DisplayMenu() {
Console.WriteLine("1) Option 1");
Console.WriteLine("2) Option 2");
Console.WriteLine("3) Option 3");
Console.WriteLine("4) Display Equation Table");
Console.Write("Enter the number that corresponds to your choice: ");
}
public static void DisplayEquation() {
Console.WriteLine("x\t5\t3x\t-2x^2\ty");
double x = 0.0;
while (x <= 2.0) {
double y = 5 + 3 * x - 2 * x * x;
Console.WriteLine($"{x:F1}\t5.0\t{3 * x:F1}\t{-2 * x * x:F1}\t{y:F1}");
x += 0.25;
}
}
}
```
In the modified `Main()` method, the user can now choose option 4 to display the equation table by invoking the `DisplayEquation()` method. The `DisplayEquation()` method uses a `while` loop to calculate the values of `y` for different values of `x`. The `F1` format specifier is used to round the output to one decimal place.
阅读全文
相关推荐
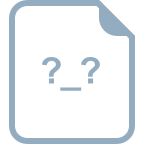
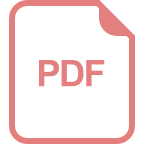
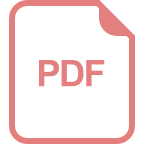
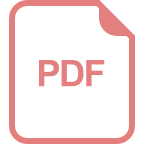
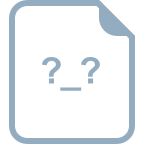
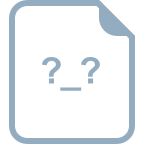
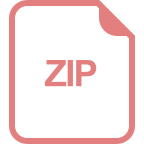
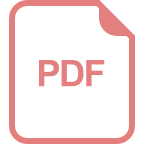
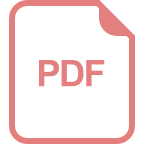
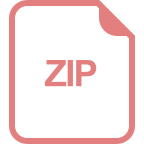
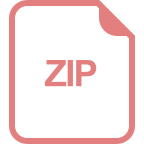
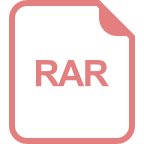
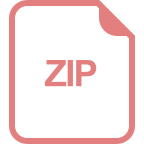
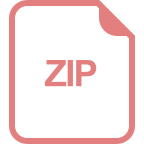
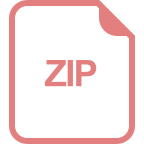
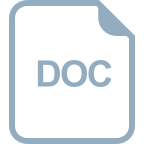
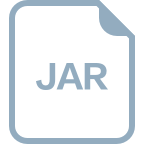
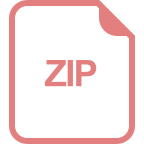