def load_rtsp(self, ip): try: self.stop() MessageBox( self.closeButton, title='Tips', text='Loading rtsp stream', time=1000, auto=True).exec_() self.det_thread.source = ip new_config = {"ip": ip} new_json = json.dumps(new_config, ensure_ascii=False, indent=2) with open('config/ip.json', 'w', encoding='utf-8') as f: f.write(new_json) self.statistic_msg('Loading rtsp:{}'.format(ip)) self.rtsp_window.close() self.det_thread.source = ip self.det_thread.is_continue = True cap = cv2.VideoCapture(ip)
时间: 2023-06-12 22:06:15 浏览: 45
这段代码看起来是一个类中的方法,主要功能是加载一个RTSP流。具体来说,它做了以下几件事情:
1. 停止当前正在运行的线程。
2. 弹出一个提示框,显示正在加载RTSP流的信息。
3. 将传入的IP地址作为新的配置信息,以JSON格式写入到文件中。
4. 关闭当前RTSP窗口。
5. 将RTSP源地址设置为传入的IP地址。
6. 将线程的`is_continue`属性设置为True,表示线程将继续执行。
7. 使用OpenCV库中的`cv2.VideoCapture`函数打开RTSP流。
需要注意的是,该代码可能存在一些问题,例如没有对`cv2.VideoCapture`函数的返回值进行检查和错误处理。另外,该方法中的一些变量和方法调用没有在代码中定义或展示,需要结合整个类的代码来理解。
相关问题
def chose_rtsp(self): self.rtsp_window = Window() config_file = 'config/ip.json' if not os.path.exists(config_file): ip = "rtsp://admin:admin888@192.168.1.67:555" new_config = {"ip": ip} new_json = json.dumps(new_config, ensure_ascii=False, indent=2) with open(config_file, 'w', encoding='utf-8') as f: f.write(new_json) else: config = json.load(open(config_file, 'r', encoding='utf-8')) ip = config['ip'] self.rtsp_window.rtspEdit.setText(ip) self.rtsp_window.show() self.rtsp_window.rtspButton.clicked.connect(lambda: self.load_rtsp(self.rtsp_window.rtspEdit.text()))
这段代码是一个函数,它的功能是让用户选择一个 RTSP 地址,然后加载这个地址的视频流。具体来说,它会先创建一个窗口对象,并将窗口显示出来。然后,它会读取一个配置文件,如果这个配置文件不存在,它会将一个默认的 RTSP 地址写入配置文件中。接着,它会从配置文件中读取 RTSP 地址,并将这个地址显示在窗口中的文本框中。最后,它会连接一个点击事件,当用户点击“确认”按钮时,会调用 `load_rtsp` 函数来加载用户选择的 RTSP 地址的视频流。
import cv2 class VideoCamera(object): url = "rtsp://admin:mliszlm79+-12@192.168.0.108:554/cam/realmonitor?channel=1&subtype=0" def __init__(self): #capturing video self.video = cv2.VideoCapture(self.url) def __del__(self): #releasing camera self.video.release()
这段代码定义了一个名为VideoCamera的对象类,其中import了cv2库用于图像处理。在类内部定义了一个常量url,被赋值为一个RTSP视频流地址,这个地址需要连接到指定的IP摄像头,并提供相应的用户名和密码。视频流地址的最后一个参数指明了要连接的视频通道序号。
相关推荐
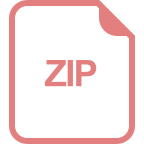











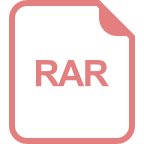
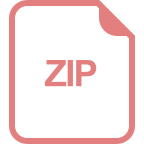