Linux open函数
时间: 2024-01-05 15:04:17 浏览: 42
在Linux系统中,open函数是用于打开或创建文件的系统调用函数。它的原型定义如下:
```c
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int open(const char *pathname, int flags);
int open(const char *pathname, int flags, mode_t mode);
```
其中,pathname参数为要打开或创建的文件路径名;flags参数为打开文件的方式及其属性标志,例如读、写、追加等;mode参数是一个八进制数,表示新创建文件的权限。
下面是一些常用的flags参数:
- O_RDONLY:只读方式打开文件。
- O_WRONLY:只写方式打开文件。
- O_RDWR:读写方式打开文件。
- O_CREAT:如果文件不存在,则创建它。
- O_TRUNC:如果文件存在并且以写方式打开,则将其截断为0。
- O_APPEND:以追加方式打开文件。
下面是一个示例,演示如何使用open函数打开文件并写入数据:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("test.txt", O_CREAT|O_WRONLY, 0666);
if (fd == -1) {
perror("open");
return -1;
}
char *msg = "Hello, world!\n";
int len = write(fd, msg, strlen(msg));
if (len == -1) {
perror("write");
close(fd);
return -1;
}
close(fd);
return 0;
}
```
在上面的代码中,我们使用open函数创建了一个名为"test.txt"的文件,并以只写方式打开它。然后,我们使用write函数向该文件写入数据,并在最后关闭文件。如果出现错误,则使用perror函数打印错误信息。
相关推荐
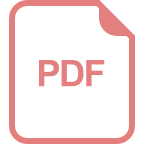
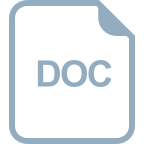
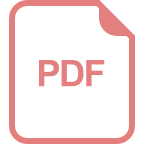











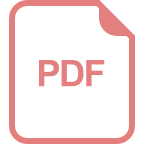
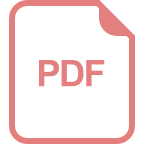
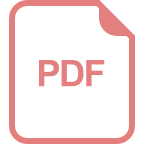
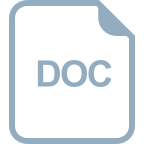