Logger logger = LoggerFactory.getLogger(joinPoint.getTarget().getClass());
时间: 2024-06-02 12:14:21 浏览: 151
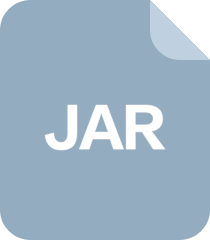
Logger.getLogger与LogFactory.getLog
This code creates a logger object using the SLF4J (Simple Logging Facade for Java) LoggerFactory class. The logger is initialized with the target class of the current join point, which is a specific point in the execution of a program (e.g. a method call). This logger can be used to log messages at various severity levels (e.g. debug, info, error) during the execution of the program, which can help with debugging and troubleshooting.
阅读全文
相关推荐
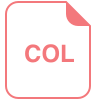
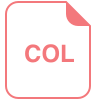



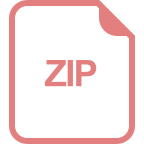
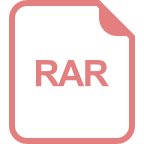
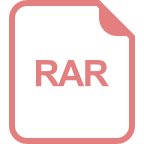
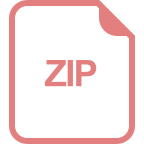
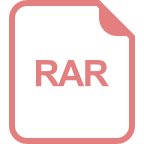
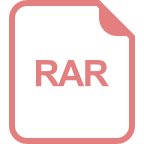
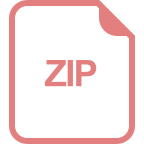
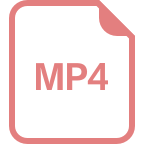
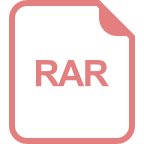