def __init__(self, input_dim=(3, 32, 32), num_filters=32, filter_size=7, hidden_dim=100, num_classes=10, weight_scale=1e-3, reg=0.0, dtype=np.float32): self.params = {} self.reg = reg self.dtype = dtype # Initialize weights and biases C, H, W = input_dim self.params['W1'] = weight_scale * np.random.randn(num_filters, C, filter_size, filter_size) self.params['b1'] = np.zeros(num_filters) self.params['W2'] = weight_scale * np.random.randn(num_filters*H*W/4, hidden_dim) self.params['b2'] = np.zeros(hidden_dim) self.params['W3'] = weight_scale * np.random.randn(hidden_dim, num_classes) self.params['b3'] = np.zeros(num_classes) for k, v in self.params.iteritems(): self.params[k] = v.astype(dtype)报错'float' object cannot be interpreted as an integer
时间: 2024-03-20 20:42:22 浏览: 13
该错误通常是由于在做整数除法时,分子或分母被当作了浮点数而不是整数。在这段代码中,错误可能出现在这一行:
```
self.params['W2'] = weight_scale * np.random.randn(num_filters*H*W/4, hidden_dim)
```
其中的`num_filters*H*W/4`是一个整数表达式,但是由于`H`和`W`的类型是整数,而`num_filters`和`4`都是浮点数常量,因此整个表达式的结果也将是浮点数。这将导致`np.random.randn()`函数的参数不是整数,从而引发异常。
为了解决这个问题,我们可以将表达式中的4改为4.0,或者使用整数除法运算符`//`,以确保表达式的结果是一个整数。例如,可以将上述代码改为:
```
self.params['W2'] = weight_scale * np.random.randn(num_filters*H*W//4, hidden_dim)
```
或者:
```
self.params['W2'] = weight_scale * np.random.randn(int(num_filters*H*W/4), hidden_dim)
```
这样就可以避免报错了。
相关问题
class ThreeLayerConvNet(object): def __init__(self, input_dim=(3, 32, 32), num_filters=32, filter_size=7, hidden_dim=100, num_classes=10, weight_scale=1e-3, reg=0.0, dtype=np.float32): self.params = {} self.reg = reg self.dtype = dtype # Initialize weights and biases C, H, W = input_dim self.params['W1'] = weight_scale * np.random.randn(num_filters, C, filter_size, filter_size) self.params['b1'] = np.zeros(num_filters) self.params['W2'] = weight_scale * np.random.randn(num_filters * H * W // 4, hidden_dim) self.params['b2'] = np.zeros(hidden_dim) self.params['W3'] = weight_scale * np.random.randn(hidden_dim, num_classes) self.params['b3'] = np.zeros(num_classes) for k, v in self.params.items(): self.params[k] = v.astype(dtype)用于mnist识别中,应该怎么修改
将这个ThreeLayerConvNet类用于MNIST数据集的识别任务,需要对其进行一些修改:
1. 输入数据的形状不同。MNIST数据集中,输入数据的形状为(1, 28, 28),所以需要将ThreeLayerConvNet的初始化函数中的input_dim改为(1, 28, 28)。
2. 输出的类别数不同。MNIST数据集中,共有10个类别,所以需要将ThreeLayerConvNet的初始化函数中的num_classes改为10。
3. 参数初始化的方式可能需要修改。由于MNIST数据集中的输入数据更小,所以可以适当减小weight_scale的值,使得参数初始化更加合适。此外,可以考虑使用其他的参数初始化方法,比如Xavier初始化。
下面是修改后的代码:
```python
class ThreeLayerConvNet(object):
def __init__(self, input_dim=(1, 28, 28), num_filters=32, filter_size=7,
hidden_dim=100, num_classes=10, weight_scale=1e-4, reg=0.0,
dtype=np.float32):
self.params = {}
self.reg = reg
self.dtype = dtype
# Initialize weights and biases
C, H, W = input_dim
self.params['W1'] = weight_scale * np.random.randn(num_filters, C, filter_size, filter_size)
self.params['b1'] = np.zeros(num_filters)
self.params['W2'] = weight_scale * np.random.randn(num_filters * H * W // 4, hidden_dim)
self.params['b2'] = np.zeros(hidden_dim)
self.params['W3'] = weight_scale * np.random.randn(hidden_dim, num_classes)
self.params['b3'] = np.zeros(num_classes)
for k, v in self.params.items():
self.params[k] = v.astype(dtype)
```
需要注意的是,这只是对ThreeLayerConvNet类进行了最基本的修改,还需要根据具体的任务进行进一步的调整和改进。
def __init__(self, input_dim=(3, 32, 32), num_filters=32, filter_size=7, hidden_dim=100, num_classes=10, weight_scale=1e-3, reg=0.0, dtype=np.float32): self.params = {} self.reg = reg self.dtype = dtype # Initialize weights and biases C, H, W = input_dim self.params['W1'] = weight_scale * np.random.randn(num_filters, C, filter_size, filter_size) self.params['b1'] = np.zeros(num_filters) self.params['W2'] = weight_scale * np.random.randn(num_filters * H * W // 4, hidden_dim) self.params['b2'] = np.zeros(hidden_dim) self.params['W3'] = weight_scale * np.random.randn(hidden_dim, num_classes) self.params['b3'] = np.zeros(num_classes) for k, v in self.params.items(): self.params[k] = v.astype(dtype)定义了W吗
这段代码定义了一个卷积神经网络的初始化函数,其中包括了一系列的超参数,如输入数据的shape、卷积核个数、卷积核大小、隐藏层神经元个数、分类数目等,以及权重初始化时的标准差、正则化系数等。
在这个初始化函数中,定义了神经网络的权重和偏置项,并将其存储在self.params字典中。其中,第一层卷积层的权重参数W1的形状为(num_filters, C, filter_size, filter_size),即(num_filters, 输入数据通道数C, 卷积核大小filter_size, 卷积核大小filter_size),第二层全连接层的权重参数W2的形状为(num_filters * H * W // 4, hidden_dim),其中H和W为输入数据的高度和宽度,//4是因为第一层卷积层使用了步幅为2的池化层,将输入数据的高度和宽度都缩小了2倍。第三层全连接层的权重参数W3的形状为(hidden_dim, num_classes),即(hidden_dim, 分类数目num_classes)。
因此,在这段代码中,确实定义了权重参数W,包括W1、W2和W3三个权重参数。
相关推荐
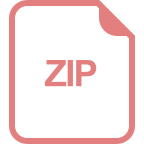













