vue2 实现 pdf文件打印,pdf浏览, pdf下载 功能
时间: 2023-10-18 22:21:21 浏览: 184
1. pdf文件打印
使用 pdf.js 库可以实现 pdf 文件的打印功能。具体实现步骤如下:
1. 在 Vue 中安装 pdf.js 库。
```
npm install pdfjs-dist
```
2. 在组件中引入 pdf.js 库,并初始化 PDFJS 对象。
```javascript
import pdfjsLib from 'pdfjs-dist/webpack'
pdfjsLib.GlobalWorkerOptions.workerSrc = 'pdfjs-dist/build/pdf.worker.js'
```
3. 加载 pdf 文件,并获取其打印页面。
```javascript
pdfjsLib.getDocument(pdfUrl).then(pdf => {
pdf.getPage(pageNumber).then(page => {
const scale = 1
const viewport = page.getViewport({ scale })
const canvas = document.createElement('canvas')
const context = canvas.getContext('2d')
canvas.height = viewport.height
canvas.width = viewport.width
page.render({ canvasContext: context, viewport }).promise.then(() => {
const imgData = canvas.toDataURL('image/png')
const printWindow = window.open('', '', 'height=600,width=800')
printWindow.document.write(`<img src="${imgData}"/>`)
printWindow.print()
})
})
})
```
2. pdf浏览
Vue 中可以使用 vue-pdf 插件实现 pdf 文件的浏览功能。具体实现步骤如下:
1. 在 Vue 中安装 vue-pdf 插件。
```
npm install vue-pdf
```
2. 在组件中使用 vue-pdf 插件,并传入 pdf 文件的 URL。
```html
<template>
<div>
<pdf :src="pdfUrl" />
</div>
</template>
<script>
import { PdfViewer as Pdf } from 'vue-pdf'
export default {
components: {
Pdf,
},
data() {
return {
pdfUrl: 'https://example.com/pdf-file.pdf',
}
},
}
</script>
```
3. pdf下载
在 Vue 中可以使用 HTML5 的 download 属性实现 pdf 文件的下载功能。具体实现步骤如下:
```html
<a :href="pdfUrl" download>Download PDF</a>
```
在上面的代码中,我们使用了 download 属性来指定文件的下载链接。当用户点击链接时,浏览器会自动下载指定的文件。
阅读全文
相关推荐
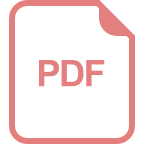
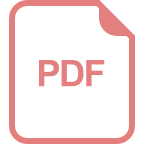
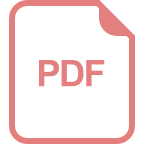















