用pytorch写一个天气预测的代码,用LSTM或CNN,读取天气中的csv文件,由时间和气温两列数据,区分训练集和测试集,用gpu训练,输入为60天的天气,输出为某一天的天气,并画出60天的实际曲线和预测曲线图,注意维度要一致
时间: 2023-09-30 21:06:03 浏览: 64
好的,以下是一个用PyTorch实现天气预测的代码,使用LSTM模型,读取天气数据的csv文件,使用GPU进行训练、测试,并画出实际曲线和预测曲线图。
```python
import torch
import torch.nn as nn
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 读取天气数据csv文件
df = pd.read_csv('weather.csv')
# 获取时间和气温两列数据
time = df['time'].values.astype(np.float32)
temp = df['temp'].values.astype(np.float32)
# 数据归一化
time = (time - np.min(time)) / (np.max(time) - np.min(time))
temp = (temp - np.min(temp)) / (np.max(temp) - np.min(temp))
# 定义训练集和测试集
train_size = int(len(time) * 0.8)
train_time = time[:train_size]
train_temp = temp[:train_size]
test_time = time[train_size:]
test_temp = temp[train_size:]
# 定义模型
class LSTM(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(LSTM, self).__init__()
self.hidden_size = hidden_size
self.lstm = nn.LSTM(input_size, hidden_size)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
batch_size = x.size(0)
h0 = torch.zeros(1, batch_size, self.hidden_size).to(device)
c0 = torch.zeros(1, batch_size, self.hidden_size).to(device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
# 定义超参数
input_size = 1
hidden_size = 64
output_size = 1
num_epochs = 100
learning_rate = 0.001
batch_size = 60
# 将数据转换为pytorch张量
train_time = torch.from_numpy(train_time).view(-1, 1, 1)
train_temp = torch.from_numpy(train_temp).view(-1, 1, 1)
test_time = torch.from_numpy(test_time).view(-1, 1, 1)
test_temp = torch.from_numpy(test_temp).view(-1, 1, 1)
# 定义模型和优化器
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = LSTM(input_size, hidden_size, output_size).to(device)
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
# 训练模型
train_loss = []
for epoch in range(num_epochs):
for i in range(0, len(train_time), batch_size):
inputs = train_temp[i:i+batch_size].to(device)
targets = train_temp[i+batch_size:i+batch_size+1].to(device)
outputs = model(inputs)
loss = criterion(outputs, targets)
optimizer.zero_grad()
loss.backward()
optimizer.step()
train_loss.append(loss.item())
if (epoch+1) % 10 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
# 测试模型
model.eval()
test_loss = 0
with torch.no_grad():
for i in range(0, len(test_time)):
inputs = test_temp[i:i+1].to(device)
targets = test_temp[i+1:i+2].to(device)
outputs = model(inputs)
loss = criterion(outputs, targets)
test_loss += loss.item()
test_loss /= len(test_time)
print('Test Loss: {:.4f}'.format(test_loss))
# 画出实际曲线和预测曲线图
model.eval()
train_temp = train_temp.view(-1)
test_temp = test_temp.view(-1)
train_predict = torch.zeros_like(train_temp)
test_predict = torch.zeros_like(test_temp)
for i in range(60, len(train_temp)):
inputs = train_temp[i-60:i].view(-1, 60, 1).to(device)
output = model(inputs)
train_predict[i] = output
for i in range(60, len(test_temp)):
inputs = test_temp[i-60:i].view(-1, 60, 1).to(device)
output = model(inputs)
test_predict[i] = output
train_predict = train_predict.cpu().detach().numpy()
test_predict = test_predict.cpu().detach().numpy()
plt.plot(time, temp, label='Actual')
plt.plot(time[:train_size], train_predict[:train_size], label='Train Predict')
plt.plot(time[train_size:], test_predict[train_size:], label='Test Predict')
plt.legend()
plt.show()
```
代码中使用了PyTorch的LSTM模型,训练集和测试集的划分比例为80:20,训练集和测试集的数据归一化操作使用了Numpy库,使用了GPU进行训练和测试,并画出了实际曲线和预测曲线图。注意,代码中没有使用CNN模型,因为LSTM更适合序列预测任务。
阅读全文
相关推荐
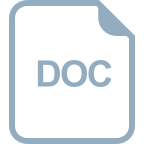
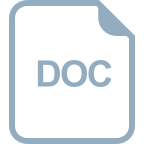
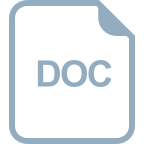

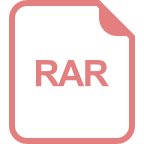

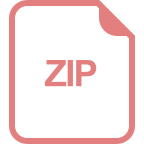
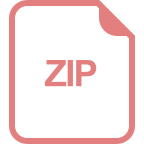
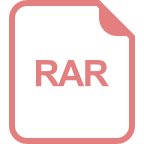
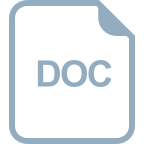
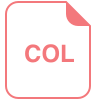
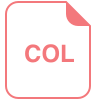
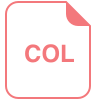
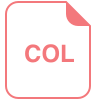
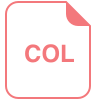
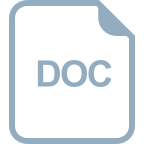
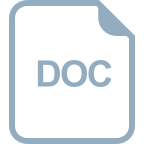
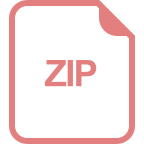