springboot图片上传下载分析
时间: 2023-06-10 13:07:39 浏览: 105
在 Spring Boot 中,实现图片上传和下载可以借助于 Spring Web 模块提供的 MultipartFile 接口和 Resource 接口。
上传图片的步骤如下:
1. 在前端页面中使用表单提交方式将图片传递到后端控制器。
2. 在后端控制器中使用 MultipartFile 接口接收图片,并将其保存到指定的路径中。
3. 返回上传成功的信息。
示例代码如下:
```java
@PostMapping("/upload")
public String uploadImage(@RequestParam("file") MultipartFile file) {
try {
// 保存图片到指定路径
String filePath = "path/to/save/image";
file.transferTo(new File(filePath));
return "Upload success!";
} catch (IOException e) {
e.printStackTrace();
return "Upload failed!";
}
}
```
下载图片的步骤如下:
1. 在前端页面中使用链接或按钮触发后端控制器。
2. 在后端控制器中将指定路径下的图片读取到 Resource 接口中,并返回该资源。
3. 在前端页面中使用 img 标签将资源展示出来。
示例代码如下:
```java
@GetMapping("/download")
public ResponseEntity<Resource> downloadImage() {
// 获取图片资源
String filePath = "path/to/save/image";
Resource resource = new FileSystemResource(filePath);
// 构建响应头
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Type", "image/png");
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
// 返回响应实体
return ResponseEntity.ok()
.headers(headers)
.contentLength(resource.contentLength())
.body(resource);
}
```
需要注意的是,在实际开发中,为了避免文件名重复等问题,应该对上传的图片进行重命名,而且要对文件的大小、格式等进行校验。
阅读全文
相关推荐
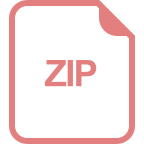
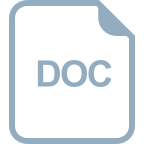
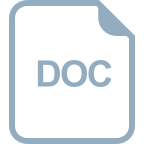
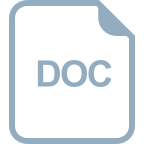
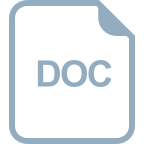
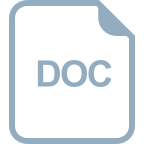
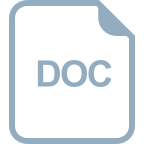
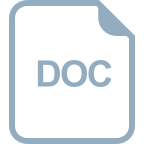
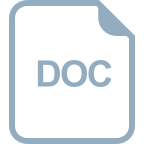
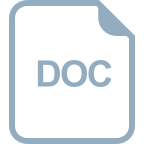
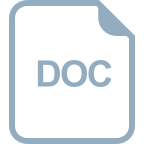
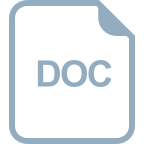
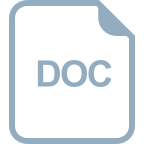
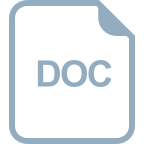
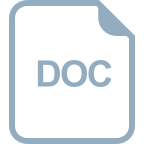
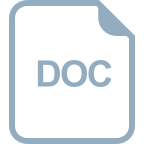