用python做一个贪吃蛇程序
时间: 2024-02-14 09:16:27 浏览: 22
好的,以下是一个基于 Python 的简单贪吃蛇程序:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
width, height = 500, 500
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("贪吃蛇")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
# 定义蛇和食物的大小
block_size = 10
# 定义字体
font = pygame.font.SysFont(None, 25)
# 定义函数,用于显示消息
def message_to_screen(msg, color):
screen_text = font.render(msg, True, color)
screen.blit(screen_text, [width/2, height/2])
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.x = width/2
self.y = height/2
self.x_change = 0
self.y_change = 0
self.body = []
self.length = 1
def draw(self):
for x,y in self.body:
pygame.draw.rect(screen, green, [x, y, block_size, block_size])
def move(self):
self.x += self.x_change
self.y += self.y_change
self.body.append((self.x, self.y))
if len(self.body) > self.length:
del self.body[0]
def change_direction(self, direction):
if direction == "LEFT":
self.x_change = -block_size
self.y_change = 0
elif direction == "RIGHT":
self.x_change = block_size
self.y_change = 0
elif direction == "UP":
self.y_change = -block_size
self.x_change = 0
elif direction == "DOWN":
self.y_change = block_size
self.x_change = 0
# 定义食物类
class Food:
def __init__(self):
self.x = round(random.randrange(0, width-block_size)/10.0)*10.0
self.y = round(random.randrange(0, height-block_size)/10.0)*10.0
def draw(self):
pygame.draw.rect(screen, red, [self.x, self.y, block_size, block_size])
# 创建
相关推荐
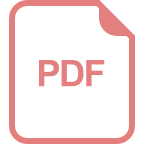
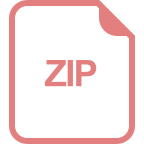
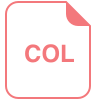
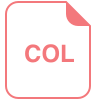
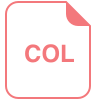
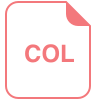








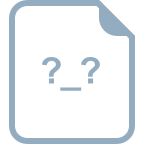
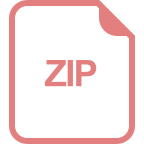