springboot项目中mybatis plus的配置
时间: 2023-09-02 20:06:25 浏览: 113
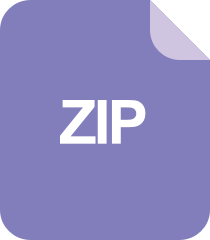
springboot + mybatis
使用MyBatis Plus可以简化MyBatis的开发,以下是在Spring Boot项目中使用MyBatis Plus的配置步骤:
1. 添加依赖
在 `pom.xml` 文件中添加 MyBatis-Plus 的依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.x.x</version>
</dependency>
```
2. 配置数据源
在 `application.properties` 文件中配置数据源:
```properties
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&useUnicode=true&characterEncoding=utf8
spring.datasource.username=root
spring.datasource.password=123456
```
3. 配置 MyBatis Plus
在 Spring Boot 中配置 MyBatis Plus 有两种方式:
方式一:使用自动配置
在 Spring Boot 中,MyBatis Plus 已经提供了自动配置的功能,你只需要在你的配置类上添加 `@MapperScan` 注解即可。
```java
@Configuration
@MapperScan("com.example.mapper") // 要扫描的Mapper接口所在的包
public class MybatisPlusConfig {
}
```
这样,MyBatis Plus 就会自动扫描指定包下的 Mapper 接口,并将其注册到 MyBatis 中。
方式二:手动配置
自动配置不适用于所有情况。如果你需要手动配置 MyBatis Plus,可以创建一个 `MybatisPlusConfig` 配置类,添加以下内容:
```java
@Configuration
public class MybatisPlusConfig {
/**
* 配置分页插件
*/
@Bean
public PaginationInterceptor paginationInterceptor() {
PaginationInterceptor paginationInterceptor = new PaginationInterceptor();
// 设置请求的页面大于最大页后操作,true调回到首页,false继续请求,默认false
paginationInterceptor.setOverflow(false);
// 设置最大单页限制数量,默认 500 条,-1 不受限制
paginationInterceptor.setLimit(500);
return paginationInterceptor;
}
}
```
在以上配置中,我们配置了 MyBatis Plus 的分页插件,使得在查询时可以进行分页。
4. 编写 Mapper 接口
使用 MyBatis Plus,你只需要编写 Mapper 接口,不需要编写对应的Mapper.xml文件,MyBatis Plus 会根据接口的方法名自动生成 SQL 语句。
```java
public interface UserMapper extends BaseMapper<User> {
}
```
在以上代码中,我们定义了一个 UserMapper 接口,并继承了 MyBatis Plus 自带的 BaseMapper 接口,这样我们就可以直接使用 MyBatis Plus 提供的增删改查方法。
5. 使用 Mapper 接口
在 Service 层中使用 `@Autowired` 注解注入 Mapper 接口。
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserById(Long id) {
return userMapper.selectById(id);
}
}
```
在以上代码中,我们在 Service 层中注入了 UserMapper 接口,并使用`selectById`方法查询用户信息。
至此,你已经完成了在 Spring Boot 中使用 MyBatis Plus 的配置。
阅读全文
相关推荐





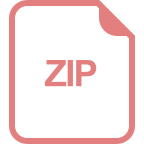
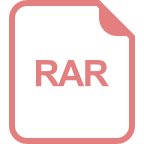







