String str = new String(buffer, 0, lenth);各参数的说明
时间: 2023-06-17 22:04:28 浏览: 154
这行代码的作用是将字节数组 `buffer` 中的前 `lenth` 个字节转换成字符串对象 `str`。
参数说明:
- `buffer`:表示要转换为字符串的字节数组。
- `0`:表示从字节数组中的第 0 个字节开始转换。
- `lenth`:表示要转换的字节个数,即转换的长度。注意,这里的长度是字节长度,而不是字符长度。如果要转换为字符长度,需要先将字节数组转换为字符串,再调用 `length()` 方法获取字符长度。
相关问题
string lenth
获取字符串长度的方法依赖于所使用的编程语言。 在大多数编程语言中,可以使用字符串的 length() 或 size() 方法来获取字符串的长度。 以下是几种编程语言的示例代码:
Java:
```
String str = "Hello World";
int len = str.length();
System.out.println("The length of the string is " + len);
```
Python:
```
str = "Hello World"
len = len(str)
print("The length of the string is", len)
```
C++:
```
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World";
int len = str.length();
cout << "The length of the string is " << len << endl;
return 0;
}
```
JavaScript:
```
let str = "Hello World";
let len = str.length;
console.log("The length of the string is " + len);
```
注意:在某些编程语言中,字符串长度可能不包括字符串末尾的空字符。
#include<iostream> #include<cstdio> #include<cstring> #include<algorithm> #include<string> using namespace std; const int maxn=5005; short int dp[2][maxn]; int n; string str1; string str2; int lenth1,lenth2; void lcs() { memset(dp,0,sizeof(dp)); lenth1=str1.length();//length返回string中字符的个数 lenth2=str2.length(); int flag=0; for(int i=0; i<=lenth1; i++) { flag=1-flag;//i-1==1-flag,i==flag for(int j=0; j<=lenth2; j++) { if(i==0||j==0) dp[flag][j]=0; else if(str1[i-1]==str2[j-1]) dp[flag][j]=dp[1-flag][j-1]+1; else dp[flag][j]=max(dp[1-flag][j],dp[flag][j-1]); } } } void swapp() { str2=str1; for(int i=0; i<n; i++) str2[i]=str1[n-1-i]; } int main() { cin>>n; cin>>str1; swapp(); lcs(); printf("%d\n",n-max(dp[0][lenth2],dp[1][lenth2])); return 0; }请添加上注释
下面是添加注释后的代码:
```c++
#include<iostream>
#include<cstdio>
#include<cstring>
#include<algorithm>
#include<string>
using namespace std;
const int maxn=5005; // 最大字符串长度
short int dp[2][maxn]; // 动态规划数组,用滚动数组优化空间复杂度
int n; // 字符串长度
string str1; // 输入的字符串
string str2; // str1反转后得到的字符串
int lenth1,lenth2; // 字符串长度
void lcs() { // 最长公共子序列算法
memset(dp,0,sizeof(dp)); // 初始化dp数组为0
lenth1=str1.length(); // 获取字符串长度
lenth2=str2.length();
int flag=0; // 滚动数组的标志位
for(int i=0; i<=lenth1; i++) // 遍历字符串的所有前缀字符
{
flag=1-flag; // 切换滚动数组标志位
for(int j=0; j<=lenth2; j++)
{
if(i==0||j==0) // 边界条件,dp[0][j]和dp[i][0]都等于0
dp[flag][j]=0;
else if(str1[i-1]==str2[j-1]) // 当前字符相等,更新dp数组
dp[flag][j]=dp[1-flag][j-1]+1;
else // 当前字符不相等,更新dp数组
dp[flag][j]=max(dp[1-flag][j],dp[flag][j-1]);
}
}
}
void swapp() { // 反转字符串的函数
str2=str1; // 将str2初始化为str1
for(int i=0; i<n; i++)
str2[i]=str1[n-1-i]; // 反转字符串
}
int main() {
cin>>n; // 输入字符串长度
cin>>str1; // 输入字符串
swapp(); // 反转字符串
lcs(); // 求解最长公共子序列
printf("%d\n",n-max(dp[0][lenth2],dp[1][lenth2])); // 输出最长回文子序列长度
return 0;
}
```
阅读全文
相关推荐
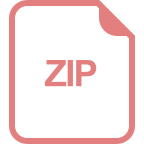
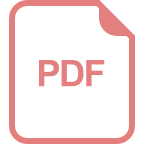
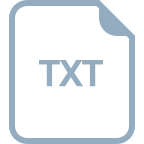













