将输入流excel导入mongodb
时间: 2023-10-08 20:08:32 浏览: 49
要将输入流excel导入MongoDB,在Java中可以使用Apache POI库来读取Excel文件,然后使用MongoDB的Java驱动程序(如Mongo Java Driver)将数据插入到MongoDB中。
以下是一个示例代码,演示了如何使用Apache POI和MongoDB的Java驱动程序将Excel数据导入MongoDB:
```java
import com.mongodb.MongoClient;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelToMongoDB {
public static void main(String[] args) {
try {
// 连接到MongoDB
MongoClient mongoClient = new MongoClient("localhost", 27017);
// 选择数据库
MongoDatabase database = mongoClient.getDatabase("your_database");
// 选择集合
MongoCollection<Document> collection = database.getCollection("your_collection");
// 读取Excel文件
FileInputStream excelFile = new FileInputStream("path/to/your/excel.xlsx");
Workbook workbook = new XSSFWorkbook(excelFile);
Sheet sheet = workbook.getSheetAt(0);
// 遍历行
for (Row row : sheet) {
Document document = new Document();
// 遍历单元格
for (Cell cell : row) {
// 根据单元格类型处理数据
switch (cell.getCellType()) {
case STRING:
document.append(cell.getAddress().toString(), cell.getStringCellValue());
break;
case NUMERIC:
document.append(cell.getAddress().toString(), cell.getNumericCellValue());
break;
// 添加其他需要处理的单元格类型
}
}
// 将文档插入MongoDB集合
collection.insertOne(document);
}
// 关闭资源
workbook.close();
excelFile.close();
mongoClient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这段代码通过连接到MongoDB,选择数据库和集合,然后使用Apache POI读取Excel文件。遍历Excel文件的每一行和每一个单元格,根据单元格的类型将数据存储到一个MongoDB文档中。最后,将文档插入MongoDB集合。
请注意,上述代码示例仅供参考,你需要根据你的实际情况进行修改。
相关推荐
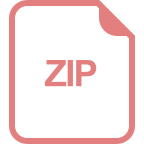
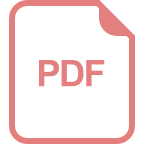














