前端如何判断一个对象是否为undefined
时间: 2024-03-27 10:37:51 浏览: 132
前端可以使用 `typeof` 操作符来判断一个对象是否为 `undefined`,如下所示:
```javascript
if (typeof someVariable === 'undefined') {
// someVariable 是 undefined
} else {
// someVariable 不是 undefined
}
```
另外,还可以使用 `===` 运算符来判断一个对象是否为 `undefined`,如下所示:
```javascript
if (someVariable === undefined) {
// someVariable 是 undefined
} else {
// someVariable 不是 undefined
}
```
需要注意的是,在判断一个未声明的变量时,使用 `typeof` 不会报错,而使用 `===` 则会报错。所以,建议在判断未声明的变量时,使用 `typeof`。
相关问题
前端取值为undefined
在前端开发中,当一个变量没有被赋值或者被赋值为 undefined 时,我们可以通过以下方式来检测它:
1. 使用 typeof 运算符,如果返回值为 "undefined",则说明变量的值为 undefined。
例如:
```
let foo;
if(typeof foo === "undefined") {
console.log("foo is undefined");
}
```
2. 使用比较运算符,如果变量的值为 undefined,则比较结果为 true。
例如:
```
let foo;
if(foo === undefined) {
console.log("foo is undefined");
}
```
需要注意的是,使用 typeof 运算符检测 null 值时,返回值也是 "object",这是一个历史遗留问题,因为在 JavaScript 的早期版本中,null 被认为是一种对象。因此,在检测 null 值时,应该使用比较运算符来进行判断。
前端 TypeError: Cannot read properties of undefined (reading 'username')
前端报错TypeError: Cannot read properties of undefined (reading 'username')是因为在代码中尝试读取一个未定义的属性'username'。这意味着该属性不存在或者其值为undefined。
要解决这个问题,可以按照以下步骤进行检查和修复:
1. 确保在访问属性之前,该属性已经被正确定义和赋值。可以在代码中使用console.log()输出相关变量或对象,检查其值是否为undefined。
2. 检查变量或对象的命名是否正确,确保没有拼写错误。
3. 如果属性是从后端返回的数据,确保后端正确地返回了该属性。可以通过查看后端接口返回的数据或者使用调试工具检查网络请求的响应数据来确认。
4. 如果属性是从后端返回的数组中的某个对象的属性,需要确保数组中的对象存在并且包含该属性。
5. 如果属性是从前端组件中获取的,可以检查组件的数据绑定或props传递是否正确。
阅读全文
相关推荐
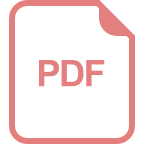
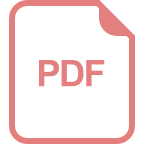
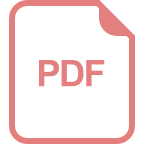
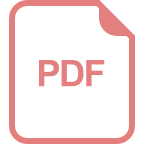




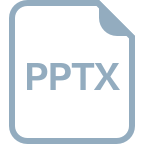
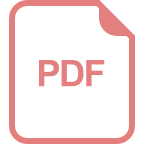
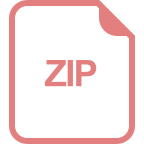
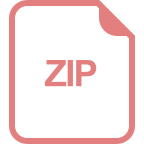




