opencv 输入一张灰度图,计算出增益与曝光时间,用于自动曝光控制算法,用于i2c操作控制相机 代码实现
时间: 2024-03-07 11:49:14 浏览: 23
以下是一个简单的代码实现,基于 OpenCV 库和 C++ 语言:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取灰度图像
Mat img = imread("test.jpg", IMREAD_GRAYSCALE);
// 计算灰度图像平均值
double mean = cv::mean(img)[0];
// 计算增益和曝光时间
int gain = 1;
int expo_time = 1;
double target_mean = 128.0; // 目标平均灰度值
if (mean < target_mean) {
// 目标灰度值比当前灰度值大,增加增益和曝光时间
while (gain < 15 && expo_time < 1000 && mean < target_mean) {
gain++;
expo_time++;
Mat tmp_img;
img.convertTo(tmp_img, -1, gain / 10.0); // 调整增益
cv::accumulateWeighted(tmp_img, img, 0.1); // 调整曝光时间
mean = cv::mean(img)[0];
}
} else {
// 目标灰度值比当前灰度值小,减小增益和曝光时间
while (gain > 1 || expo_time > 1 && mean > target_mean) {
gain--;
expo_time--;
Mat tmp_img;
img.convertTo(tmp_img, -1, gain / 10.0); // 调整增益
cv::accumulateWeighted(tmp_img, img, 0.1); // 调整曝光时间
mean = cv::mean(img)[0];
}
}
// 输出结果
cout << "Gain: " << gain << endl;
cout << "Exposure time: " << expo_time << endl;
return 0;
}
```
这里的算法基于反馈控制原理,不断调整增益和曝光时间,使得灰度图像的平均值逐渐接近目标灰度值。代码中使用了 `convertTo` 函数进行增益调整,使用了 `accumulateWeighted` 函数进行曝光时间调整。
相关推荐
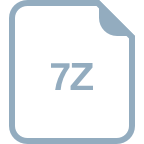
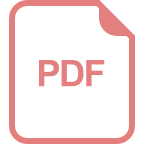














