用c语言写出任意输入一行字符串保存在一维字符数组中,判断里面数字字符的个数
时间: 2024-02-09 10:11:58 浏览: 27
可以使用C语言中的字符数组和循环结构来实现此功能。下面是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
int count = 0;
printf("请输入一行字符串:");
fgets(str, 100, stdin);
for (int i = 0; i < strlen(str); i++) {
// 判断字符是否为数字
if (str[i] >= '0' && str[i] <= '9') {
count++;
}
}
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
解释一下代码:
1. 首先定义了一个长度为100的字符数组 `str`,用于保存输入的字符串。
2. 然后使用 `fgets()` 函数从标准输入中获取用户输入的一行字符串,最多读取100个字符。
3. 接下来使用一个 `for` 循环遍历字符串中的每一个字符,如果该字符是数字字符,则将计数器 `count` 值加1。
4. 最后输出数字字符的个数。
需要注意的是,上述代码只能统计ASCII码表中的数字字符,如果要支持更多的字符集,需要对字符编码进行相应的处理。
相关问题
用c语言输入一行字符统计其中每个英文字母的个数存放到一维数组中
以下是一个示例程序,可以实现输入一行字符统计其中每个英文字母的个数,并将结果存放到一维数组中:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_LENGTH 1000 // 输入字符串的最大长度
#define NUM_LETTERS 26 // 英文字母的数量
int main() {
char str[MAX_LENGTH];
int counts[NUM_LETTERS] = {0}; // 初始值全部为0
printf("请输入一行字符:\n");
fgets(str, MAX_LENGTH, stdin); // 读取一行字符
// 统计每个英文字母的个数
for (int i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i])) { // 判断是否为英文字母
int index = tolower(str[i]) - 'a'; // 转换为小写字母后计算下标
counts[index]++;
}
}
// 输出结果
for (int i = 0; i < NUM_LETTERS; i++) {
if (counts[i] > 0) {
printf("%c: %d\n", 'a' + i, counts[i]);
}
}
return 0;
}
```
程序中使用了几个关键函数:
- `fgets()`:读取一行字符,包括换行符。
- `isalpha()`:判断一个字符是否为英文字母。
- `tolower()`:将大写字母转换为小写字母。
- 数组下标计算:将小写字母转换为对应的数字下标,例如 `a` 对应下标 `0`,`b` 对应下标 `1`,以此类推。
需要注意的是,程序中只统计了小写字母的个数,如果需要统计大写字母的个数,可以在计算下标时先使用 `tolower()` 函数转换为小写字母。
C语言,统计二维字符数组中字母,数字,空格,其他字符的个数,用循环,输入每行的字符串
可以使用两层循环,第一层循环遍历输入的每一行字符串,第二层循环遍历当前行字符串的每一个字符,然后使用if-else语句进行分类统计。
具体代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char arr[100][100];
int n, letter_count, digit_count, space_count, other_count;
printf("Enter the number of lines: ");
scanf("%d", &n);
getchar(); // 吃掉输入数字后的回车符
for (int i = 0; i < n; i++) {
printf("Enter string %d: ", i+1);
gets(arr[i]); // 获取当前行字符串
}
for (int i = 0; i < n; i++) {
letter_count = digit_count = space_count = other_count = 0; // 每次循环前先清零
for (int j = 0; j < strlen(arr[i]); j++) {
if (arr[i][j] >= 'a' && arr[i][j] <= 'z' || arr[i][j] >= 'A' && arr[i][j] <= 'Z') {
letter_count++;
} else if (arr[i][j] >= '0' && arr[i][j] <= '9') {
digit_count++;
} else if (arr[i][j] == ' ') {
space_count++;
} else {
other_count++;
}
}
printf("Line %d:\n", i+1);
printf("Letter count: %d\n", letter_count);
printf("Digit count: %d\n", digit_count);
printf("Space count: %d\n", space_count);
printf("Other count: %d\n", other_count);
}
return 0;
}
```
示例输入:
```
Enter the number of lines: 3
Enter string 1: Hello World!
Enter string 2: 12345 abcdefg
Enter string 3: ^&$#)
```
输出结果:
```
Line 1:
Letter count: 10
Digit count: 0
Space count: 1
Other count: 1
Line 2:
Letter count: 7
Digit count: 5
Space count: 1
Other count: 0
Line 3:
Letter count: 0
Digit count: 0
Space count: 0
Other count: 6
```
相关推荐
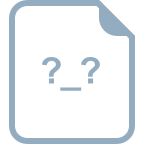
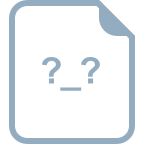












