使用java代码静态扫描指定springboot项目中使用的mysql地址,redis地址
时间: 2023-08-31 14:25:47 浏览: 145
### 回答1:
要实现静态扫描指定的Spring Boot项目中使用的MySQL地址和Redis地址,可以使用以下代码:
```
import org.springframework.boot.autoconfigure.jdbc.DataSourceProperties;
import org.springframework.boot.autoconfigure.data.redis.RedisProperties;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Application {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext("com.example");
// 扫描MySQL地址
DataSourceProperties dataSourceProperties = context.getBean(DataSourceProperties.class);
System.out.println("MySQL地址: " + dataSourceProperties.getUrl());
// 扫描Redis地址
RedisProperties redisProperties = context.getBean(RedisProperties.class);
System.out.println("Redis地址: " + redisProperties.getHost() + ":" + redisProperties.getPort());
}
}
```
这个代码使用Spring的ApplicationContext从指定的包中扫描Bean,获取到数据源和Redis的配置信息,然后输出它们的地址。需要注意的是,这个代码需要在已经运行的Spring Boot应用程序中运行,因为ApplicationContext需要在应用程序中进行初始化。
### 回答2:
使用Java代码静态扫描指定Spring Boot项目中使用的MySQL地址和Redis地址,可以通过以下步骤实现:
1. 导入必要的依赖项和类:
- 借助Spring Boot的IoC容器,需要导入`spring-context`相关依赖。
- 导入`org.springframework.boot.autoconfigure.jdbc.DataSourceProperties`类,用于获取MySQL地址。
- 导入`org.springframework.boot.autoconfigure.data.redis.RedisProperties`类,用于获取Redis地址。
2. 创建一个扫描类`ScanUtils`,其中包含以下两个方法:
- `getMySQLAddress()`:获取MySQL地址。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.jdbc.DataSourceProperties;
import org.springframework.stereotype.Component;
@Component
public class ScanUtils {
@Autowired
private DataSourceProperties dataSourceProperties;
public String getMySQLAddress() {
return dataSourceProperties.getUrl();
}
}
```
- `getRedisAddress()`:获取Redis地址。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.data.redis.RedisProperties;
import org.springframework.stereotype.Component;
@Component
public class ScanUtils {
@Autowired
private RedisProperties redisProperties;
public String getRedisAddress() {
return redisProperties.getHost() + ":" + redisProperties.getPort();
}
}
```
3. 在Spring Boot项目的启动类中注入`ScanUtils`并使用它获取MySQL地址和Redis地址。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class YourApplication {
@Autowired
private ScanUtils scanUtils;
public static void main(String[] args) {
SpringApplication.run(YourApplication.class, args);
}
public void yourMethod() {
// 获取MySQL地址
String mySQLAddress = scanUtils.getMySQLAddress();
System.out.println("MySQL地址:" + mySQLAddress);
// 获取Redis地址
String redisAddress = scanUtils.getRedisAddress();
System.out.println("Redis地址:" + redisAddress);
}
}
```
通过以上步骤,我们可以使用Java代码静态扫描指定的Spring Boot项目中使用的MySQL地址和Redis地址,并将它们打印出来。
### 回答3:
要使用Java代码进行静态扫描指定Spring Boot项目中使用的MySQL地址和Redis地址,可以使用以下步骤:
1. 引入相关的依赖:
在项目的pom.xml文件中添加MySQL和Redis相关依赖,例如:
```
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>${mysql.version}</version>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>${redis.version}</version>
</dependency>
```
2. 编写扫描代码:
创建一个Java类,例如`ProjectScanner`,编写用于扫描MySQL地址和Redis地址的代码。可以使用Java的反射机制来扫描Spring Boot项目中的注解和配置类。
例如,对于MySQL地址,可以使用以下代码:
```java
public class ProjectScanner {
public static void main(String[] args) {
ClassPathScanningCandidateComponentProvider scanner = new ClassPathScanningCandidateComponentProvider(false);
scanner.addIncludeFilter(new AnnotationTypeFilter(Configuration.class));
Set<BeanDefinition> beanDefinitions = scanner.findCandidateComponents("com.example.springboot");
for (BeanDefinition beanDefinition : beanDefinitions) {
try {
String className = beanDefinition.getBeanClassName();
Class<?> clazz = Class.forName(className);
if (clazz.isAnnotationPresent(EnableAutoConfiguration.class)) {
EnableAutoConfiguration annotation = clazz.getAnnotation(EnableAutoConfiguration.class);
String[] excluded = annotation.exclude();
for (String exclude : excluded) {
if (exclude.contains("MySQL")) {
// 处理MySQL地址逻辑
// ...
}
}
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
}
```
对于Redis地址,可以使用类似的方式进行扫描。
3. 替换`com.example.springboot`为你指定的Spring Boot项目的包名。
4. 编译并运行上述代码,将会输出在Spring Boot项目中使用的MySQL地址和Redis地址信息。
注意:
- 以上代码仅仅是演示如何通过扫描获取MySQL地址和Redis地址的思路,具体实现需要根据实际情况做相应的调整和处理。
- 代码示例中使用了MySQL和Redis的示例代码,实际项目中可能使用不同的数据库和缓存组件,需要相应地进行修改。
希望能对你有所帮助!
阅读全文
相关推荐
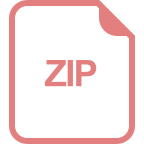
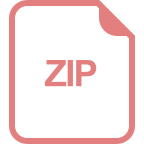
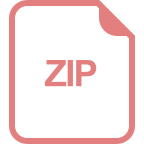
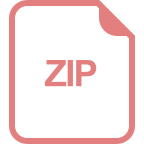
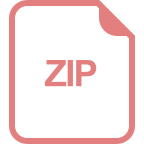
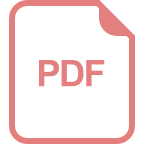
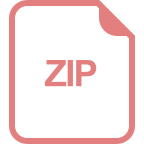
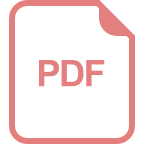
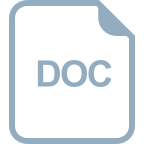
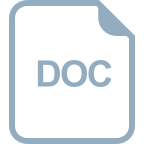
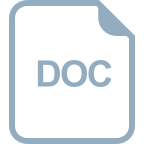
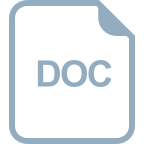
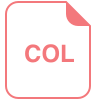
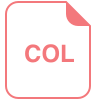
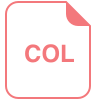
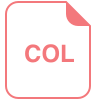
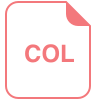