mixture of experts
时间: 2023-04-19 07:02:44 浏览: 274
混合专家模型(Mixture of Experts)是一种机器学习模型,它由多个专家模型组成,每个专家模型对应不同的输入空间。混合专家模型通过对输入进行分类,将输入分配给不同的专家模型进行处理,最终将各个专家模型的输出进行加权平均得到最终的输出结果。混合专家模型在处理复杂的非线性问题时具有很好的性能,被广泛应用于语音识别、图像处理、自然语言处理等领域。
相关问题
function [model, loglikHist] = mixexpFit(X, y, nmix, varargin) %% Fit a mixture of experts model via MLE/MAP using EM % If the response y is real-valued, we use linear regression experts. % If the response y is categorical, we use logistic regression experts. % % Inputs % % X - X(i, :) is the ith case, i.e. data is of size n-by-d % y - y(i) can be real valued or in {1..C} % nmix - the number of mixture components to use % % % Optional inputs % EMargs - cell array. See emAlgo. (Default {}) % fixmix - if true, mixing weights are constants independent of x % (default false) % nclasses - needed if not all labels are present in y % (default nunique(y)) % preproc - a struct, passed to preprocessorApplyToTtrain % By default, this adds ones and standardizes % gatingFitArgs - cell array, default {'lambda', 0.001} % expertFitArgs - cell array, default {'lambda', 0.001} % % Outputs % % A structure - see mixExpCreate for field descriptions % loglikHist - a record of the log likelihood at each EM iteration. %% % This file is from pmtk3.googlecode.com pp = preprocessorCreate('addOnes', true, 'standardizeX', true); [EMargs, fixmix, nclasses, preproc, gatingFitArgs, expertFitArgs] = ... process_options(varargin, ... 'EMargs', {}, 'fixmix', false, 'nclasses', [], 'preproc', pp, ... 'gatingFitArgs', {'lambda', 0.001}, ... 'expertFitArgs', {'lambda', 0.001}); [preproc, X] = preprocessorApplyToTrain(preproc, X); % We use k=1:nmix to index mixture components % and c=1:C to index output classes [N,D] = size(X); %X = standardize(X); %X = [ones(N,1) X]; %D = D+1; if isequal(y, round(y)) model.classifier = true; if isempty(nclasses) nclasses = numel(unique(y)); end else model.classifier = false; nclasses = 1; end data.X = X; data.y = y; model.nmix = nmix; model.nclasses = nclasses; model.D = D; model.preproc = preproc; model.expertFitArgs = expertFitArgs; model.gatingFitArgs = gatingFitArgs; model.fixmix = fixmix; model.modelType = 'mixexp'; [model, loglikHist] = emAlgo(model, data, @initFn, @estep, @mstep, ... EMargs{:}); end
这段代码是一个用于拟合混合专家模型的函数 `mixexpFit`。
该函数的输入参数包括:
- `X`: 输入变量的数据,大小为 n-by-d,其中 n 是样本数,d 是输入变量的维度;
- `y`: 输出变量的数据,可以是实值或者分类变量,大小为 n-by-1;
- `nmix`: 混合成分的数量;
- `varargin`: 可选参数,包括 EMargs、fixmix、nclasses、preproc、gatingFitArgs 和 expertFitArgs 等。
函数的输出包括:
- `model`: 拟合后得到的混合专家模型,是一个结构体;
- `loglikHist`: EM 算法迭代过程中的对数似然值记录。
在函数内部,首先对输入参数进行一些预处理操作,如标准化输入变量 `X`、添加偏置项等。
然后,根据输出变量 `y` 的类型(实值或分类变量),设置相应的模型类型和输出类别个数。
接下来,调用 EM 算法的函数 `emAlgo` 进行参数估计。其中,需要传入一些函数句柄,包括初始化函数 `initFn`、E 步函数 `estep` 和 M 步函数 `mstep`。还可以传入 EM 算法的参数 `EMargs`。
最后,将拟合得到的模型和对数似然值记录返回。
这段代码的作用是拟合一个混合专家模型,可以适用于实值或分类问题。模型的参数估计使用了 EM 算法。
如果还有其他问题,欢迎提问!
function varargout = mixexpPredict(model, X) %% Predict using mixture of experts model % If the response y is real-valued, we return % [mu, sigma2, post, muk, sigma2k] = mixexpPredict(model, X) % mu(i) = E[y | X(i,:)] % sigma2(i) = var[y | X(i,:)] % weights(i,k) = p(expert = k | X(i,:) % muk(i) = E[y | X(i,:), expert k] % sigma2k(i) = var[y | X(i,:), expert k] % % If the response y is categorical, we return % [yhat, prob] = mixexpPredict(model, X) % yhat(i) = argmax p(y|X(i,:)) % prob(i,c) = p(y=c|X(i,:)) % This file is from pmtk3.googlecode.com [N,D] = size(X); %X = standardize(X); %X = [ones(N,1) X]; if isfield(model, 'preproc') [X] = preprocessorApplyToTest(model.preproc, X); end K = model.nmix; if model.fixmix weights = repmat(model.mixweights, N, 1); else weights = softmaxPmtk(X*model.Wq); % weights(n,q) end if model.classifier % implemented by JoAnne Ting prob = zeros(N, size(model.Wy,2)); yhat_k = zeros(N, model.Nclasses, K); for k = 1:K yhat_k(:,:,k) = softmaxPmtk(X*model.Wy(:,:,k)); % Weighted vote prob = prob + yhat_k(:,:,k) .* repmat(weights(:,k), 1, size(model.Wy,2)); end yhat = maxidx(prob, [], 2); varargout{1} = yhat; varargout{2} = prob; else % mean of a mixture model is given by % E[x] = sum_k pik muk %mu = sum(weights .* (X*model.Wy), 2); % variance of a mixture model is given by % sum_k pi_k [Sigmak + muk*muk'] - E[x] E[x]' muk = zeros(N,K); vk = zeros(N,K); mu = zeros(N,1); v = zeros(N,1); for k=1:K muk(:,k) = X*model.Wy(:,k); mu = mu + weights(:,k) .* muk(:,k); vk(:,k) = model.sigma2(k); v = v + weights(:,k) .* (vk(:,k) + muk(:,k).^2); end v = v-mu.^2; varargout{1} = mu; varargout{2} = v; varargout{3} = weights; varargout{4} = muk; varargout{5} = vk; end end
这是一个用于预测混合专家模型的函数。该函数可以根据输入的模型和数据,返回预测结果。如果目标变量 y 是实值型的,函数会返回预测的均值、方差以及后验概率。如果目标变量 y 是分类型的,函数会返回预测的类别以及类别的概率。
函数首先对输入数据 X 进行预处理(如果有预处理步骤),然后根据模型参数计算混合权重 weights。如果模型是分类器,则根据权重和类别权重参数计算类别概率,并选取概率最大的类别作为预测结果。如果模型不是分类器,则根据权重和模型参数计算预测的均值和方差,并返回混合权重、均值和方差。
该函数是从 pmtk3.googlecode.com 中获取的。
阅读全文
相关推荐
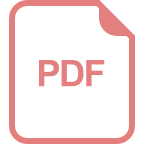
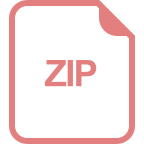
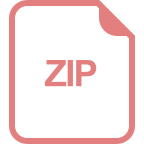
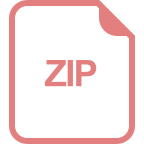
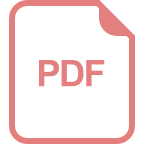
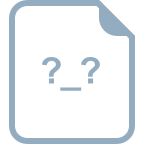
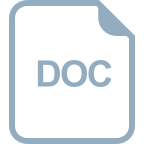




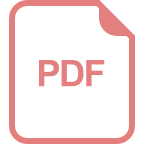
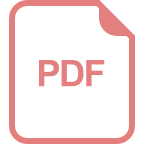
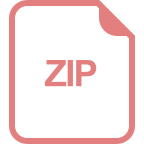
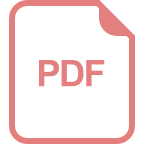