python导入数据行数列数
时间: 2023-07-26 17:18:36 浏览: 98
要导入数据的行数和列数,可以使用Python中的pandas库。具体步骤如下:
1. 导入pandas库:
```python
import pandas as pd
```
2. 使用pandas的read_csv函数读取数据:
```python
data = pd.read_csv('data.csv')
```
其中,data.csv是要读取的数据文件名。
3. 查看数据的行数和列数:
```python
row_num, col_num = data.shape
print("行数:", row_num)
print("列数:", col_num)
```
这里使用了pandas的shape属性,它会返回一个元组,第一个元素是行数,第二个元素是列数。
相关问题
导入数据文件:设计代码导入指定数据文件。查看导入数据:设计代码获得导入数据的行数、列数
以及数据内容。具体实现可以使用Python中的pandas库,读取数据文件并将其转化为数据框,然后获取数据框的行数、列数以及数据内容。
示例代码如下:
```python
import pandas as pd
# 导入数据文件
data_file = 'data.csv'
data = pd.read_csv(data_file)
# 获取行数和列数
num_rows = data.shape[0]
num_cols = data.shape[1]
print('数据文件共有{}行,{}列'.format(num_rows, num_cols))
# 查看数据内容
print(data.head())
```
其中,`data_file`是数据文件的路径,可以根据实际情况进行修改。`pd.read_csv`函数可以读取csv格式的数据文件,也可以根据实际情况选择其他格式的读取函数。`data.shape`可以获取数据框的形状,即行数和列数。`data.head()`可以查看数据框的前几行数据,默认为前5行。
xlwd 获取sheet的行数和列数
要使用xlrd库获取Excel表格中Sheet的行数和列数,您可以按照以下步骤进行操作:
首先,导入xlrd库:
```python
import xlrd
```
接下来,打开Excel文件并选择要操作的Sheet:
```python
workbook = xlrd.open_workbook('your_file.xls') # 替换为您的Excel文件名
sheet = workbook.sheet_by_index(0) # 选择要操作的Sheet,这里选择第一个Sheet,索引从0开始
```
然后,使用`nrows`属性获取Sheet的行数,使用`ncols`属性获取Sheet的列数:
```python
rows = sheet.nrows # 获取行数
cols = sheet.ncols # 获取列数
```
最后,您可以打印行数和列数,或者根据需要进行其他操作:
```python
print("行数:", rows)
print("列数:", cols)
```
请确保您已经安装了xlrd库,并将代码中的文件名替换为您要操作的实际文件名。
相关推荐
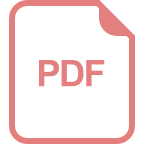
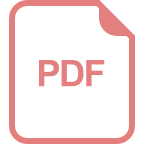
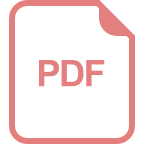
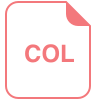











