string转time_t
时间: 2024-05-09 20:21:44 浏览: 112
好的,这是一个比较简单的问题。可以使用C++标准库中的函数来实现。你可以使用strptime函数将一个string类型的时间字符串转换为tm结构体类型,然后再使用mktime函数将tm结构体类型转换为time_t类型。具体的代码如下:
```
#include <ctime>
#include <string>
time_t string_to_time_t(const std::string& time_str)
{
struct tm tm_time = {0};
strptime(time_str.c_str(), "%Y-%m-%d %H:%M:%S", &tm_time);
return mktime(&tm_time);
}
```
这个函数将输入的时间字符串解析为格式为"年-月-日 时:分:秒"的格式,并将其转换为time_t类型的时间戳。你可以根据实际需要修改输入的时间格式。
相关问题
c++ time_t 转 string
在C++中可以使用std::to_string函数将time_t类型的时间戳转换为string类型。具体实现如下:
```c++
#include <iostream>
#include <ctime>
#include <string>
int main() {
std::time_t timestamp = std::time(nullptr);
std::string time_string = std::to_string(timestamp);
std::cout << "当前时间戳为:" << time_string << std::endl;
return 0;
}
```
其中,std::to_string函数可以将time_t类型的时间戳转换为字符串类型。在输出时,将其作为字符串输出即可。
c++ 类型转换 time_point_t 转换为 stding
你可以使用 `std::chrono::duration_cast` 函数来将 `time_point_t` 类型转换为 `std::string` 类型。首先,你需要确定要转换的时间单位,比如秒、毫秒、微秒等等。然后,你可以使用 `time_since_epoch` 函数获取时间点相对于纪元的持续时间,将其转换为所需的时间单位,最后使用 `std::to_string` 函数将其转换为字符串表示。下面是一个示例代码:
```cpp
#include <chrono>
#include <string>
using time_point_t = std::chrono::system_clock::time_point;
std::string timePointToString(time_point_t timePoint) {
auto duration = timePoint.time_since_epoch();
// 假设要转换为秒
auto seconds = std::chrono::duration_cast<std::chrono::seconds>(duration);
return std::to_string(seconds.count());
}
int main() {
time_point_t currentTime = std::chrono::system_clock::now();
std::string currentTimeString = timePointToString(currentTime);
// 使用 currentTimeString 进行操作或输出
return 0;
}
```
在上面的示例中,`timePointToString` 函数将 `time_point_t` 类型的时间点转换为以秒为单位的字符串表示。你可以根据需要修改函数中的类型转换部分,以实现不同的时间单位转换。
阅读全文
相关推荐
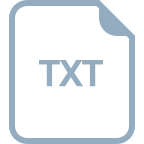
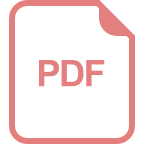













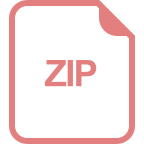