java统计一段话中各个单词数目
时间: 2023-10-23 17:23:50 浏览: 39
可以使用Java中的HashMap来实现统计单词数目的功能。具体实现步骤如下:
1. 将输入的字符串按照空格进行分割,得到一个字符串数组。
2. 遍历该字符串数组,将每个字符串作为key存入HashMap中,并将其对应的value值初始为1。
3. 对于已存入HashMap中的字符串,将其对应的value值加1。
4. 遍历完所有字符串后,HashMap中的每个key对应的value值即为该单词在字符串中出现的次数。
下面是示例代码:
```java
import java.util.HashMap;
public class WordCount {
public static void main(String[] args) {
String str = "This is a test sentence. This sentence is for testing.";
String[] words = str.split(" ");
HashMap<String, Integer> map = new HashMap<String, Integer>();
for (String word : words) {
if (map.containsKey(word)) {
int count = map.get(word);
map.put(word, count+1);
} else {
map.put(word, 1);
}
}
for (String key : map.keySet()) {
System.out.println(key + ": " + map.get(key));
}
}
}
```
输出结果为:
```
This: 2
is: 2
a: 1
test: 1
sentence.: 1
sentence: 1
for: 1
testing.: 1
```
相关推荐
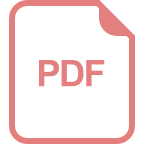














