生成JAVA教学管理系统代码
时间: 2023-07-21 14:10:44 浏览: 51
以下是一个简单的JAVA教学管理系统代码,包含了学生信息的增删改查和成绩管理功能。代码中使用了MySQL数据库来存储学生信息和成绩数据,使用了JDBC技术来进行数据库操作。请注意替换代码中的数据库连接信息和表名等参数。
```java
import java.sql.*;
public class StudentManagementSystem {
// 数据库连接信息,请根据实际情况修改
private static final String JDBC_URL = "jdbc:mysql://localhost:3306/test";
private static final String JDBC_USERNAME = "root";
private static final String JDBC_PASSWORD = "123456";
// 数据库表名,请根据实际情况修改
private static final String STUDENT_TABLE = "students";
private static final String SCORE_TABLE = "scores";
// 查询学生信息
public static void queryStudent(String id) {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(JDBC_URL, JDBC_USERNAME, JDBC_PASSWORD);
String sql = "SELECT * FROM " + STUDENT_TABLE + " WHERE id = ?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, id);
rs = stmt.executeQuery();
if (rs.next()) {
String name = rs.getString("name");
int age = rs.getInt("age");
String gender = rs.getString("gender");
System.out.println("学号:" + id + " 姓名:" + name + " 年龄:" + age + " 性别:" + gender);
} else {
System.out.println("学号为 " + id + " 的学生不存在");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 添加学生信息
public static void addStudent(String id, String name, int age, String gender) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DriverManager.getConnection(JDBC_URL, JDBC_USERNAME, JDBC_PASSWORD);
String sql = "INSERT INTO " + STUDENT_TABLE + " (id, name, age, gender) VALUES (?, ?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, id);
stmt.setString(2, name);
stmt.setInt(3, age);
stmt.setString(4, gender);
int result = stmt.executeUpdate();
if (result == 1) {
System.out.println("添加学生信息成功");
} else {
System.out.println("添加学生信息失败");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 修改学生信息
public static void updateStudent(String id, String name, int age, String gender) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DriverManager.getConnection(JDBC_URL, JDBC_USERNAME, JDBC_PASSWORD);
String sql = "UPDATE " + STUDENT_TABLE + " SET name = ?, age = ?, gender = ? WHERE id = ?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, name);
stmt.setInt(2, age);
stmt.setString(3, gender);
stmt.setString(4, id);
int result = stmt.executeUpdate();
if (result == 1) {
System.out.println("修改学生信息成功");
} else {
System.out.println("修改学生信息失败");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 删除学生信息
public static void deleteStudent(String id) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DriverManager.getConnection(JDBC_URL, JDBC_USERNAME, JDBC_PASSWORD);
String sql = "DELETE FROM " + STUDENT_TABLE + " WHERE id = ?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, id);
int result = stmt.executeUpdate();
if (result == 1) {
System.out.println("删除学生信息成功");
} else {
System.out.println("删除学生信息失败");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 输入学生成绩
public static void inputScore(String id, int score) {
Connection conn = null;
PreparedStatement stmt = null;
try {
conn = DriverManager.getConnection(JDBC_URL, JDBC_USERNAME, JDBC_PASSWORD);
String sql = "INSERT INTO " + SCORE_TABLE + " (id, score) VALUES (?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, id);
stmt.setInt(2, score);
int result = stmt.executeUpdate();
if (result == 1) {
System.out.println("输入学生成绩成功");
} else {
System.out.println("输入学生成绩失败");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 查询成绩排行榜
public static void queryScoreRank() {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(JDBC_URL, JDBC_USERNAME, JDBC_PASSWORD);
String sql = "SELECT s.id, st.name, s.score FROM " + SCORE_TABLE + " s JOIN " + STUDENT_TABLE + " st ON s.id = st.id ORDER BY s.score DESC";
stmt = conn.prepareStatement(sql);
rs = stmt.executeQuery();
int rank = 1;
while (rs.next()) {
String id = rs.getString("id");
String name = rs.getString("name");
int score = rs.getInt("score");
System.out.println("排名:" + rank + " 学号:" + id + " 姓名:" + name + " 成绩:" + score);
rank++;
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 查询成绩分布图
public static void queryScoreDistribution() {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection(JDBC_URL, JDBC_USERNAME, JDBC_PASSWORD);
String sql = "SELECT COUNT(*) AS cnt, FLOOR(score / 10) * 10 AS range FROM " + SCORE_TABLE + " GROUP BY FLOOR(score / 10) * 10 ORDER BY range";
stmt = conn.prepareStatement(sql);
rs = stmt.executeQuery();
while (rs.next()) {
int cnt = rs.getInt("cnt");
int range = rs.getInt("range");
System.out.println(range + "-" + (range + 9) + "分:" + cnt + "人");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
// 查询学生信息
queryStudent("001");
// 添加学生信息
addStudent("002", "张三", 20, "男");
// 修改学生信息
updateStudent("002", "张三", 21, "男");
// 删除学生信息
deleteStudent("002");
// 输入学生成绩
inputScore("001", 80);
// 查询成绩排行榜
queryScoreRank();
// 查询成绩分布图
queryScoreDistribution();
}
}
```
希望以上代码能够对您有所帮助。如果您有其他问题或者需要更详细的解答,请随时提出。
相关推荐
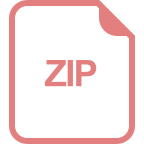
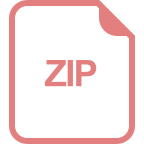
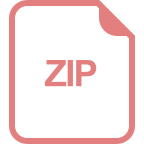














