获取文件名工具类c++代码
时间: 2023-09-24 15:10:49 浏览: 130
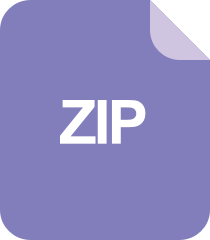
C++获取某路径下所有文件名称代码
以下是一个获取文件名的工具类的 C++ 代码示例,其中包含了两种方法:
```c++
#include <iostream>
#include <string>
#include <algorithm>
class FilenameUtils {
public:
// 方法 1:使用 std::string::find_last_of() 和 substr() 函数
static std::string getFileName(const std::string& path) {
size_t found = path.find_last_of("/\\");
return path.substr(found + 1);
}
// 方法 2:使用 std::string::find_last_of() 和 std::string::erase() 函数
static std::string getFileName2(const std::string& path) {
size_t found = path.find_last_of("/\\");
if (found != std::string::npos) {
return path.erase(0, found + 1);
}
return "";
}
};
int main() {
std::string path = "/path/to/file.txt";
std::string filename = FilenameUtils::getFileName(path);
std::cout << "Filename: " << filename << std::endl;
std::string path2 = "C:\\path\\to\\file.txt";
std::string filename2 = FilenameUtils::getFileName2(path2);
std::cout << "Filename: " << filename2 << std::endl;
return 0;
}
```
这个工具类包含了两种方法:
- 方法 1:使用 `std::string::find_last_of()` 和 `substr()` 函数来获取最后一个路径分隔符后面的子字符串,即文件名。
- 方法 2:使用 `std::string::find_last_of()` 和 `std::string::erase()` 函数,先删除路径分隔符及其前面的字符串,再返回剩余的字符串作为文件名。
你可以根据自己的需求选用其中的一种方法实现文件名的获取。
阅读全文
相关推荐
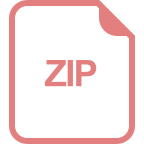
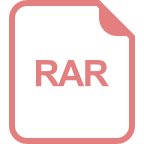
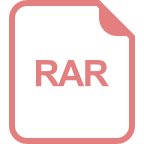
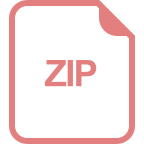
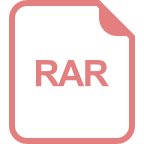
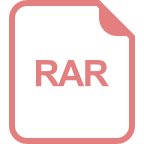
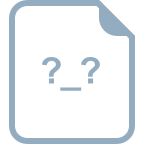
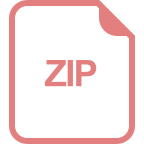
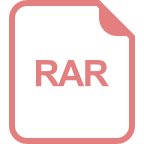
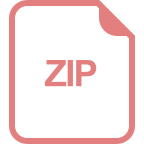
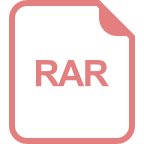
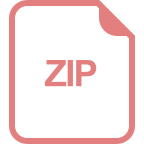
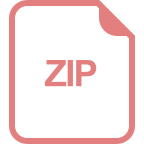
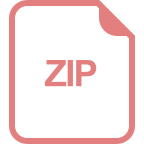
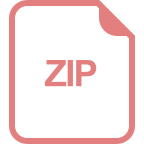
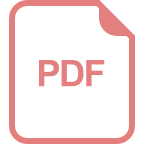