package total.test; import total.date.*; public class AppTest { public static void main(String [] args) { Point [] point = new Point[7]; for(int i=0;i<point.length;i++) { point[i] = new Point(); point[i].setX(i); point[i].setY(10); } Animal [] left = new Animal[3]; Animal [] right = new Animal[3]; for(int i =0;i<left.length;i++ ){ left[i] = new LeftAnimal(); left[i].setName("猫"+i); left[i].setAtPoint(point[i]); left[i].setAllCanAtPoint(point); } for(int i =0;i<right.length;i++ ){ right[i] = new RightAnimal(); right[i].setName("狗"+i); right[i].setAtPoint(point[4+i]); right[i].setAllCanAtPoint(point); } input(point); if(right[0].move()) input(point); if(left[2].move()) input(point); if(left[1].move()) input(point); if(right[0].move()) input(point); if(right[1].move()) input(point); if(right[2].move()) input(point); if(left[2].move()) input(point); if(left[1].move()) input(point); if(left[0].move()) input(point); if(right[0].move()) input(point); if(right[1].move()) input(point); if(right[2].move()) input(point); if(left[1].move()) input(point); if(left[0].move()) input(point); if(right[2].move()) input(point); } static void input(Point [] point){ for(int i=0;i<point.length;i++){ Animal animal=point[i].getAtPointAnimal(); if(animal!=null) System.out.print(animal.getName()); else System.out.print(" "); } System.out.println(); } }帮我注释代码
时间: 2024-04-15 22:31:02 浏览: 71
Sure! Here's the commented version of your code:
```java
package total.test;
import total.date.*;
public class AppTest {
public static void main(String[] args) {
Point[] point = new Point[7]; // 创建一个长度为7的Point数组
for (int i = 0; i < point.length; i++) {
point[i] = new Point(); // 实例化每个数组元素
point[i].setX(i); // 设置每个点的X坐标为i
point[i].setY(10); // 设置每个点的Y坐标为10
}
Animal[] left = new Animal[3]; // 创建一个长度为3的左边动物数组
Animal[] right = new Animal[3]; // 创建一个长度为3的右边动物数组
for (int i = 0; i < left.length; i++) {
left[i] = new LeftAnimal(); // 实例化左边动物对象
left[i].setName("猫" + i); // 设置左边动物的名称为"猫i"
left[i].setAtPoint(point[i]); // 设置左边动物所在的点为point[i]
left[i].setAllCanAtPoint(point); // 设置左边动物可以到达的所有点为point数组
}
for (int i = 0; i < right.length; i++) {
right[i] = new RightAnimal(); // 实例化右边动物对象
right[i].setName("狗" + i); // 设置右边动物的名称为"狗i"
right[i].setAtPoint(point[4 + i]); // 设置右边动物所在的点为point[4+i]
right[i].setAllCanAtPoint(point); // 设置右边动物可以到达的所有点为point数组
}
input(point); // 调用input方法打印当前动物所在的点
// 以下是一系列移动动物并打印当前动物所在的点的操作
if (right[0].move())
input(point);
if (left[2].move())
input(point);
if (left[1].move())
input(point);
if (right[0].move())
input(point);
if (right[1].move())
input(point);
if (right[2].move())
input(point);
if (left[2].move())
input(point);
if (left[1].move())
input(point);
if (left[0].move())
input(point);
if (right[0].move())
input(point);
if (right[1].move())
input(point);
if (right[2].move())
input(point);
if (left[1].move())
input(point);
if (left[0].move())
input(point);
if (right[2].move())
input(point);
}
static void input(Point[] point) {
for (int i = 0; i < point.length; i++) {
Animal animal = point[i].getAtPointAnimal(); // 获取每个点上的动物对象
if (animal != null)
System.out.print(animal.getName()); // 如果动物对象不为空,则打印动物名称
else
System.out.print(" "); // 如果动物对象为空,则打印空格
}
System.out.println();
}
}
```
I have added comments to explain each section of the code. Let me know if you have any further questions!
阅读全文
相关推荐










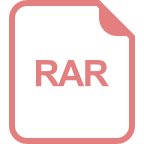






