使用JavaSwing开发的代码,利用io流文件存储数据,可以实现ATM系统的基本注册登录、转账、查询、存取款业务 功能要求: 1.用户登录注册 2.用户存款 3.用户查询余额(查询账户余额和操作记录) 4.用户取款 5.用户转账功能
时间: 2024-03-09 18:49:13 浏览: 13
感谢您的提问,我会尽力回答!
首先,为了实现ATM系统的基本功能,我们需要先设计出数据结构和存储方式。一种常见的方法是使用Java对象和IO流,将用户信息和操作记录存储在文件中。下面是一个简单的用户类和操作记录类的示例:
```
public class User implements Serializable {
private String username;
private String password;
private double balance;
// getter and setter methods
}
public class Transaction implements Serializable {
private String type; // deposit, withdraw, or transfer
private double amount;
private String from;
private String to;
private Date date;
// getter and setter methods
}
```
接下来是主界面的设计和实现,我们可以使用JavaSwing库来进行界面布局和事件处理。下面是一个简单的界面示例:
```
public class ATMGUI extends JFrame {
private User currentUser;
private List<Transaction> transactions;
// GUI components
// ...
public ATMGUI() {
// initialization code
// ...
}
private void login() {
// handle login event
// ...
}
private void register() {
// handle register event
// ...
}
private void deposit() {
// handle deposit event
// ...
}
private void withdraw() {
// handle withdraw event
// ...
}
private void transfer() {
// handle transfer event
// ...
}
private void showBalance() {
// show account balance and transaction history
// ...
}
}
```
接下来是具体的业务逻辑实现,包括用户登录注册、存取款、转账和查询余额等功能。这里我们可以使用Java IO流来读写文件,并且对于每个操作都需要更新用户信息和操作记录。下面是一个简单的业务逻辑示例:
```
public class ATM {
private List<User> users;
private List<Transaction> transactions;
// IO operations
// ...
public ATM() {
// initialization code
// ...
}
public boolean login(String username, String password) {
// find the user by username and verify the password
// ...
}
public boolean register(String username, String password) {
// check if the username is available and add a new user
// ...
}
public boolean deposit(double amount) {
// update the user balance and add a new transaction
// ...
}
public boolean withdraw(double amount) {
// check if the balance is sufficient and update the user balance and add a new transaction
// ...
}
public boolean transfer(String toUsername, double amount) {
// check if the toUser exists and the balance is sufficient, update both user balances and add a new transaction
// ...
}
public User getCurrentUser() {
// return the current user object
// ...
}
public List<Transaction> getTransactions() {
// return the transaction history for the current user
// ...
}
}
```
以上是一个简单的ATM系统实现的示例,当然还有很多细节需要考虑和完善。希望能对您有所帮助!
相关推荐
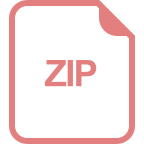
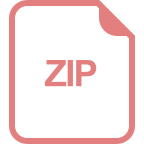
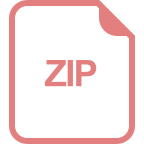














