使用C语言写一个发送AT指令代码框架,要易于添加AT指令,每一个AT指令都需要处理回复的消息
时间: 2024-10-11 10:05:30 浏览: 26
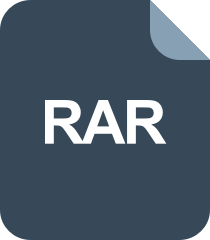
gprs.rar_at GPRS _at指令 封装_gprs mc45_mc45
在C语言中,编写一个用于发送AT指令并处理回复消息的基础框架,通常会包含以下几个关键部分:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 宏定义串口通信函数原型
#define SERIAL_COMM(command) sendSerialCommand(command, AT_RESPONSE_LENGTH)
// 发送AT命令到串口,并接收固定长度的回复
void sendSerialCommand(char* command, int response_length) {
// 打开串口或假设已经打开
FILE* serial_port = fopen("/dev/ttyS0", "w"); // 替换为实际的串口路径
if (serial_port == NULL) {
perror("Failed to open serial port");
return;
}
// 发送AT命令
fputs(command, serial_port);
fflush(serial_port);
// 接收回复,这里仅做示例,实际应用需要适当解析
char reply[response_length + 1];
fgets(reply, response_length + 1, serial_port);
// 关闭串口
fclose(serial_port);
// 处理回复(例如检查错误码或提取信息)
process_response(reply);
}
// 模拟处理回复的函数,可以根据实际需求修改
void process_response(char* reply) {
if (strlen(reply) > 0 && strcmp(reply, "+OK") == 0) {
printf("Received OK for command: %s\n", reply);
} else {
printf("Error in response: %s\n", reply);
}
}
// 主函数,演示如何使用
int main() {
// 添加AT指令示例
const char* atCommands[] = {
"AT", // 查询设备状态
"AT+CMGF=1", // 设置短信模式
"AT+CPIN?" // 检查SIM卡PIN
};
const int num_commands = sizeof(atCommands) / sizeof(atCommands[0]);
for (int i = 0; i < num_commands; ++i) {
SERIAL_COMM(atCommands[i]);
// 或者添加更多处理逻辑,如等待一段时间后发送下一个AT指令
}
return 0;
}
```
阅读全文
相关推荐
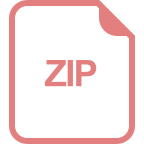
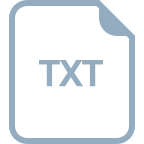
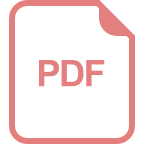
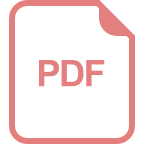
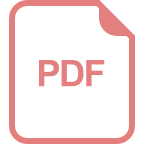
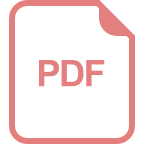
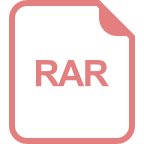
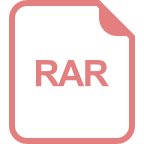
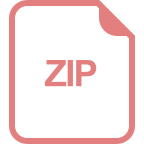
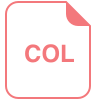
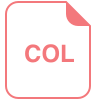
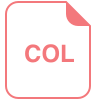
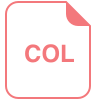
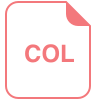
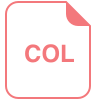
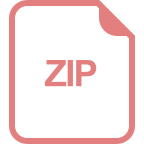