Echarts实现3d环形进度条
时间: 2024-01-17 22:04:08 浏览: 251
Echarts提供了一个3D柱状图(bar3D)的组件,我们可以利用这个组件实现一个3D环形进度条。
具体实现步骤如下:
1. 安装Echarts
```bash
npm install echarts --save
```
2. 引入Echarts库
```html
<script src="https://cdn.jsdelivr.net/npm/echarts/dist/echarts.min.js"></script>
```
3. 创建一个容器
```html
<div id="chart-container"></div>
```
4. 初始化Echarts实例
```js
var chart = echarts.init(document.getElementById('chart-container'));
```
5. 配置Echarts参数
```js
var option = {
xAxis3D: {
type: 'category',
data: ['进度'],
},
yAxis3D: {
type: 'category',
data: [''],
},
zAxis3D: {
type: 'value',
},
grid3D: {
boxWidth: 200,
boxDepth: 80,
viewControl: {
autoRotate: true,
},
},
series: [
{
type: 'bar3D',
data: [[0, 0, 0]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
{
type: 'bar3D',
data: [[0, 0, 1]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
{
type: 'bar3D',
data: [[0, 0, 2]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
{
type: 'bar3D',
data: [[0, 0, 3]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
],
};
```
6. 渲染Echarts图表
```js
chart.setOption(option);
```
7. 动态更新进度条
```js
function updateProgress(progress) {
chart.setOption({
series: [
{
data: [[0, 0, progress]],
},
],
});
}
```
完整的代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>3D环形进度条</title>
<script src="https://cdn.jsdelivr.net/npm/echarts/dist/echarts.min.js"></script>
</head>
<body>
<div id="chart-container"></div>
<script>
var chart = echarts.init(document.getElementById('chart-container'));
var option = {
xAxis3D: {
type: 'category',
data: ['进度'],
},
yAxis3D: {
type: 'category',
data: [''],
},
zAxis3D: {
type: 'value',
},
grid3D: {
boxWidth: 200,
boxDepth: 80,
viewControl: {
autoRotate: true,
},
},
series: [
{
type: 'bar3D',
data: [[0, 0, 0]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
{
type: 'bar3D',
data: [[0, 0, 1]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
{
type: 'bar3D',
data: [[0, 0, 2]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
{
type: 'bar3D',
data: [[0, 0, 3]],
shading: 'color',
itemStyle: {
opacity: 0.6,
},
},
],
};
chart.setOption(option);
function updateProgress(progress) {
chart.setOption({
series: [
{
data: [[0, 0, progress]],
},
],
});
}
// test
setTimeout(function () {
updateProgress(1);
}, 1000);
setTimeout(function () {
updateProgress(2);
}, 2000);
setTimeout(function () {
updateProgress(3);
}, 3000);
setTimeout(function () {
updateProgress(0);
}, 4000);
</script>
</body>
</html>
```
这样就实现了一个简单的3D环形进度条。
阅读全文
相关推荐
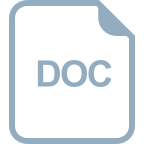
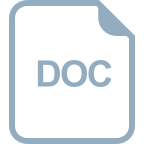
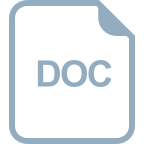



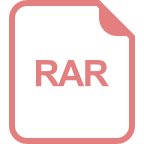
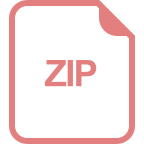
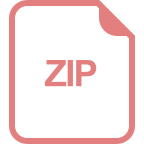
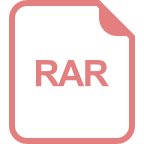
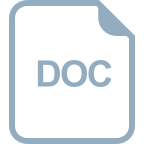



