java语言寻找一组按SOAP接口协议进行通信的公开服务,例如天气预报服务,查阅这些服务的接口格式,为其中的服务操作构造单元测试、执行测试,并验证测试结果是否正确
时间: 2023-05-25 07:05:01 浏览: 85
以下是一个寻找以SOAP协议通信的公开服务示例:
我们将使用天气预报服务进行测试。该服务的WSDL地址为:http://www.webservicex.net/globalweather.asmx?WSDL
接口格式如下:
- GetCitiesByCountry:根据国家名称获取该国家下的所有城市列表。
- GetWeather:根据城市名称和国家名称获取天气预报信息。
接下来,我们将为上述服务操作构造单元测试和执行测试,并验证测试结果是否正确。
1. 创建一个Java项目,并在pom.xml中添加以下依赖项:
```
<dependency>
<groupId>com.sun.xml.ws</groupId>
<<artifactId>jaxws-rt</artifactId>
<version>2.3.0</version>
</dependency>
```
2. 创建一个服务类,并在其中定义两个方法:getCitiesByCountry和getWeather。
```
@Service
@WebService(endpointInterface = "com.example.service.GlobalWeatherService")
public class GlobalWeatherServiceImpl implements GlobalWeatherService {
@Override
public String getCitiesByCountry(String country) {
// TODO: 实现获取城市列表的逻辑
return null;
}
@Override
public String getWeather(String country, String city) {
// TODO: 实现获取天气预报信息的逻辑
return null;
}
}
```
3. 在服务类中实现getCitiesByCountry和getWeather方法。
```
@Override
public String getCitiesByCountry(String country) {
try {
GlobalWeather service = new GlobalWeather();
GlobalWeatherSoap port = service.getGlobalWeatherSoap12();
return port.getCitiesByCountry(country);
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
@Override
public String getWeather(String country, String city) {
try {
GlobalWeather service = new GlobalWeather();
GlobalWeatherSoap port = service.getGlobalWeatherSoap12();
return port.getWeather(city, country);
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
```
4. 创建一个单元测试类,并编写测试用例。
```
public class GlobalWeatherServiceTest {
private static final String COUNTRY_NAME = "United States";
private static final String CITY_NAME = "New York";
@Test
public void testGetCitiesByCountry() {
GlobalWeatherServiceImpl service = new GlobalWeatherServiceImpl();
String citiesXML = service.getCitiesByCountry(COUNTRY_NAME);
assertThat(citiesXML).isNotNull().isNotEmpty();
}
@Test
public void testGetWeather() {
GlobalWeatherServiceImpl service = new GlobalWeatherServiceImpl();
String weatherXML = service.getWeather(COUNTRY_NAME, CITY_NAME);
assertThat(weatherXML).isNotNull().isNotEmpty();
}
}
```
5. 执行测试,并验证结果是否正确。
```
public class GlobalWeatherServiceTest {
private static final String COUNTRY_NAME = "United States";
private static final String CITY_NAME = "New York";
@Test
public void testGetCitiesByCountry() {
GlobalWeatherServiceImpl service = new GlobalWeatherServiceImpl();
String citiesXML = service.getCitiesByCountry(COUNTRY_NAME);
assertThat(citiesXML).isNotNull().isNotEmpty();
}
@Test
public void testGetWeather() {
GlobalWeatherServiceImpl service = new GlobalWeatherServiceImpl();
String weatherXML = service.getWeather(COUNTRY_NAME, CITY_NAME);
assertThat(weatherXML).isNotNull().isNotEmpty();
// 解析XML并验证返回结果
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(new InputSource(new StringReader(weatherXML)));
doc.getDocumentElement().normalize();
// 从XML中获取天气预报信息
NodeList nList = doc.getElementsByTagName("Temperature");
String temperature = nList.item(0).getTextContent();
assertThat(temperature).isNotNull().isNotEmpty();
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
```
以上示例演示了如何寻找以SOAP协议通信的公开服务,并编写单元测试来测试其接口功能。您可以使用类似的方法来测试其他公开服务。
阅读全文
相关推荐
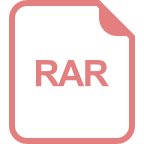
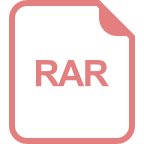
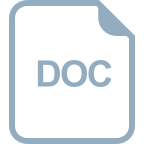
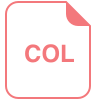
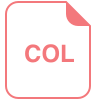
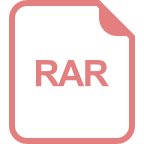
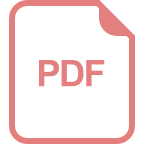
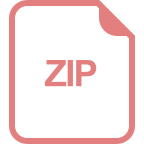