VC6.0读取xml文件
时间: 2025-01-06 22:36:31 浏览: 2
### 如何在 Visual C++ 6.0 中读取 XML 文件
为了在 Visual C++ 6.0 中读取 XML 文件,可以利用 Microsoft 提供的 MSXML 库。MSXML 支持通过智能指针简化编程模型[^1]。
#### 创建项目并设置环境
首先,在 Visual C++ 6.0 中创建一个新的控制台应用程序项目。接着配置项目的属性以支持 COM 组件:
- 添加对 `msxml6.dll` 的引用;
- 设置预处理器定义 `_WIN32_WINNT=0x0501` 或更高版本来启用必要的 Windows 功能。
#### 初始化 COM 库
由于 MSXML 是基于 COM 技术构建的库,在使用之前需要初始化 COM 环境:
```cpp
#include <windows.h>
#include <comdef.h> // For _bstr_t and other COM types
#include <msxml6.h>
int main() {
CoInitialize(NULL); // Initialize the COM library on the current thread
try {
// Your code here
} catch (_com_error &e) {
printf("Error: %s\n", (char*)e.Description());
}
CoUninitialize(); // Uninitialize the COM library
}
```
#### 加载和解析 XML 文档
下面展示了一个完整的例子,说明如何加载一个本地磁盘上的 XML 文件,并打印根节点名称及其第一个子元素的内容:
```cpp
#include <iostream>
using namespace std;
// Include necessary headers for working with MSXML
#import "C:\Program Files\Common Files\System\ado\msxml6.dll" \
named_guids rename("EOF","adEOF")
void LoadAndParseXml(const char* filePath) {
HRESULT hr;
// Create an instance of the DOMDocument object using smart pointers
_COM_SMARTPTR_TYPEDEF(IXMLDOMDocument, __uuidof(IXMLDOMDocument));
IXMLDOMDocumentPtr pDoc(__uuidof(DOMDocument));
// Set properties to ensure proper parsing behavior
pDoc->async = VARIANT_FALSE; // Synchronous loading
pDoc->validateOnParse = VARIANT_FALSE;// Do not validate against DTD or schema
// Load the specified file into memory as a document tree structure
VARIANT varPath;
varPath.vt = VT_BSTR;
varPath.bstrVal = _bstr_t(filePath);
if (!pDoc->load(varPath)) throw new exception();
// Accessing elements within the parsed data structure
cout << L"Root element name is '" << pDoc->documentElement->nodeName << "'." << endl;
// Get first child node under root element
IXMLDOMNodePtr pNode = pDoc->documentElement->firstChild;
while(pNode != NULL && pNode->nodeType != NODE_ELEMENT){
pNode = pNode->nextSibling;
}
wcout << L"The content of its first sub-element is '"
<< static_cast<IXMLDOMText*>(pNode)->text << "'.";
}
int main(){
CoInitialize(NULL);
try{
const wchar_t *path=L"C:\\example.xml"; // Replace this path accordingly
LoadAndParseXml(path);
}catch(_com_error& e){
cerr<<"An error occurred:"<<e.ErrorMessage()<<endl;
}
CoUninitialize();
return 0;
}
```
此程序展示了基本的操作流程,包括打开文件、访问文档结构以及提取特定的信息片段。对于更复杂的查询需求,则可能需要用到 XPath 表达式或其他高级特性。
阅读全文
相关推荐





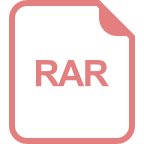



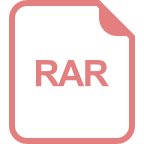
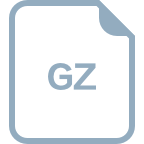


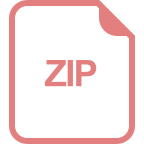

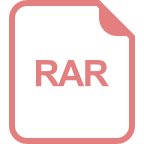

