BluetoothGattCallback 数据传输 实例代码
时间: 2024-10-19 18:12:58 浏览: 9
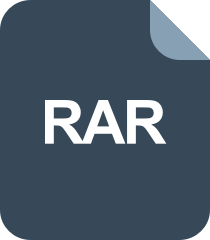
蓝牙4.0经典代码实例
蓝牙低功耗(GATT)回调接口`BluetoothGattCallback`是Android Beacon Library或类似库中用于处理蓝牙设备数据传输的核心部分。这个回调接口通常在连接到蓝牙设备后设置,以便在接收服务、特征值读写等操作成功或失败时得到通知。
以下是一个简单的例子,展示了如何创建并使用`BluetoothGattCallback`实例:
```java
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothGatt;
import android.bluetooth.BluetoothGattCallback;
import android.bluetooth.BluetoothGattCharacteristic;
import android.bluetooth.BluetoothGattService;
// 创建回调接口实现
class CustomGattCallback extends BluetoothGattCallback {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothGatt.STATE_CONNECTED) {
// 连接成功,可以开始读写数据
gatt.discoverServices();
} else {
// 关联状态改变,例如断开连接
handleConnectionError(status);
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 获取所有服务
List<BluetoothGattService> services = gatt.getServices();
for (BluetoothGattService service : services) {
// 发现感兴趣的特性并注册读写事件
gatt.readCharacteristic(service.getCharacteristics().get(0));
}
} else {
handleDiscoveryError(status);
}
}
private void handleConnectionError(int status) {
// 处理错误,如超时、权限问题等
}
private void handleDiscoveryError(int status) {
// 处理服务发现错误
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
// 读取特性值后处理结果
if (status == BluetoothGatt.GATT_SUCCESS) {
byte[] data = characteristic.getValue();
// ...解析并处理数据
} else {
handleReadError(status);
}
}
// 其他回调方法如onCharacteristicWrite, onDescriptorWrite等,可以根据需要添加处理逻辑
private void handleReadError(int status) {
// 处理读取特性值时的错误
}
}
// 主动创建并设置回调
BluetoothGatt gatt = yourBluetoothAdapter.connect(device, new CustomGattCallback());
```
在这个示例中,当蓝牙连接建立或服务发现完成时,会有相应的回调执行。在读取特定特征值时,如果操作成功,会获取到该值并进一步处理;如果有错误,则会在对应的错误处理方法中捕获异常。
阅读全文
相关推荐
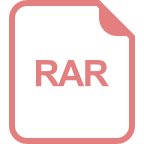
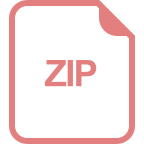
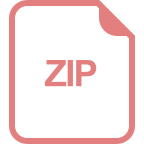
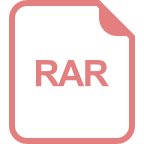
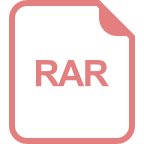
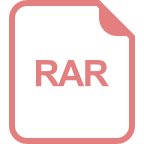
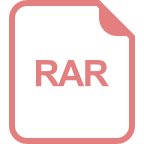
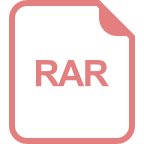
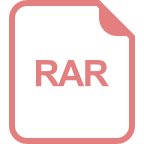
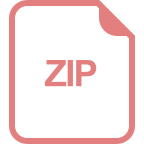
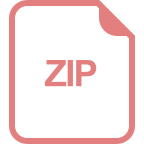
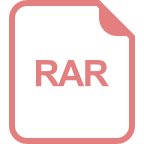
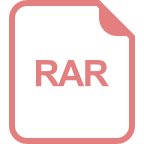
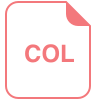
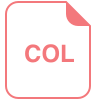
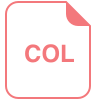
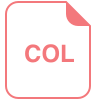

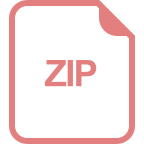