c语言创建一个单向链表
时间: 2024-09-11 13:00:38 浏览: 23
在C语言中,创建一个单向链表通常涉及以下几个步骤:
1. **定义节点结构**:
首先,你需要定义一个节点结构体,包含数据域(如`data`)和指向下一个节点的指针(如`next`)。
```c
struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
};
```
2. **创建链表头节点**:
创建链表的第一步通常是创建一个空的头节点(如果有的话),用于表示链表的开始。
```c
struct Node* head = NULL;
```
3. **添加节点**:
你可以定义一个函数来插入新节点到链表中,例如:
- 插入到链表的末尾:
```c
void append(struct Node** head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
struct Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
```
- 插入到指定位置:
```c
void insertAtPosition(struct Node** head, int data, int position) {
// ... 实现代码,检查边界条件、分配内存并插入等
}
```
4. **遍历链表**:
可以编写一个函数来打印链表的所有元素:
```c
void printList(struct Node* node) {
while (node != NULL) {
printf("%d -> ", node->data);
node = node->next;
}
printf("NULL\n");
}
```
5. **释放内存**:
当不再需要链表时,记得释放所有节点的内存以避免内存泄漏:
```c
void destroyList(struct Node** head) {
struct Node* current = *head;
struct Node* next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
*head = NULL;
}
```
相关推荐
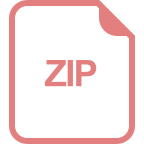
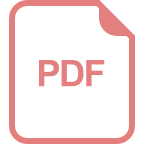
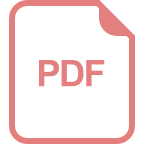
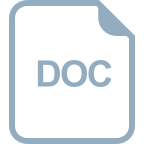
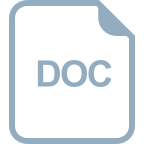
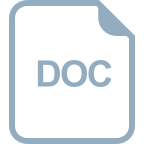
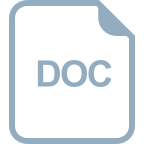
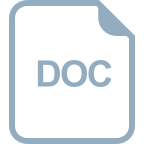
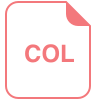
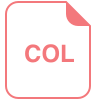
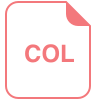
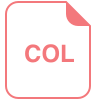
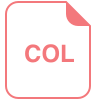




