Android c++ 打印函数调用栈
时间: 2024-02-10 17:09:18 浏览: 174
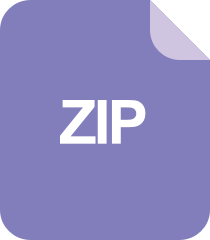
Android调用C++函数
在Android C++开发中,可以使用以下方法来打印函数调用栈:
1. 使用backtrace函数:backtrace函数可以获取当前线程的函数调用栈信息。需要包含头文件<execinfo.h>。示例代码如下:
```cpp
#include <execinfo.h>
#include <stdio.h>
void printStackTrace() {
void* callstack[128];
int frames = backtrace(callstack, 128);
char** strs = backtrace_symbols(callstack, frames);
if (strs == nullptr) {
perror("backtrace_symbols");
return;
}
for (int i = 0; i < frames; ++i) {
printf("%s\n", strs[i]);
}
free(strs);
}
```
2. 使用libunwind库:libunwind是一个用于获取函数调用栈信息的库。需要在Android.mk文件中添加libunwind库的链接。示例代码如下:
```cpp
#include <libunwind.h>
#include <stdio.h>
void printStackTrace() {
unw_cursor_t cursor;
unw_context_t context;
unw_getcontext(&context);
unw_init_local(&cursor, &context);
while (unw_step(&cursor) > 0) {
unw_word_t offset, pc;
char symbol[256];
unw_get_reg(&cursor, UNW_REG_IP, &pc);
if (unw_get_proc_name(&cursor, symbol, sizeof(symbol), &offset) == 0) {
printf("[%p] %s + %lu\n", reinterpret_cast<void*>(pc), symbol, offset);
} else {
printf("[%p] <unknown>\n", reinterpret_cast<void*>(pc));
}
}
}
```
这两种方法都可以在C++代码中调用,用于打印当前线程的函数调用栈信息。需要注意的是,这些方法只能在调试模式下使用,不应该在正式发布的应用中使用。
阅读全文
相关推荐
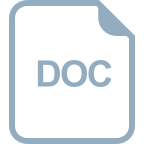
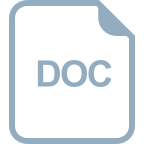
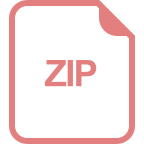
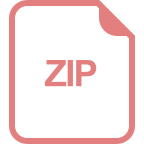
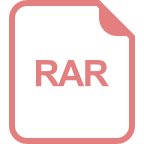

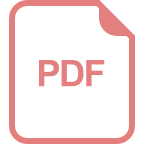
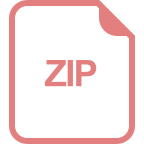
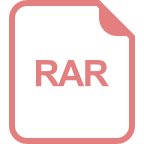
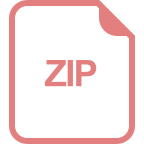
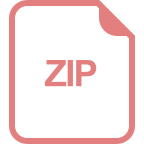
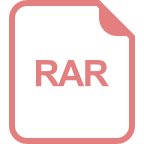
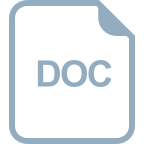


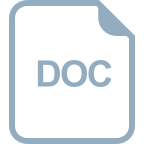
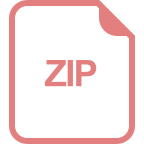
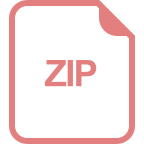