Winform中在form1中有button1,radiobutton1,radiobutton2,radiobutton3,form2中有txtbox,现在点击button1进入form2中,并且选择一个radiobutton,form1跳转到form2,在txtbox中显示出选择的radiobutton的text,用委托和多线程来写
时间: 2024-04-16 13:27:25 浏览: 112
在这个问题中,我们可以使用委托和多线程来实现Form1跳转到Form2并显示所选择的RadioButton的Text。以下是实现这段代码的示例:
在Form1中:
```csharp
public partial class Form1 : Form
{
private Form2 form2;
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
form2 = new Form2();
form2.RadioButtonSelected += OnRadioButtonSelected;
// 使用多线程打开Form2
Thread thread = new Thread(new ThreadStart(OpenForm2));
thread.Start();
}
private void OpenForm2()
{
Application.Run(form2);
}
private void OnRadioButtonSelected(string text)
{
// 在Form2中选择的RadioButton的Text
string selectedText = text;
// 在Form1中更新UI控件(txtbox)
if (textBox1.InvokeRequired)
{
textBox1.Invoke(new MethodInvoker(delegate { textBox1.Text = selectedText; }));
}
else
{
textBox1.Text = selectedText;
}
}
}
```
在Form2中:
```csharp
public partial class Form2 : Form
{
public delegate void RadioButtonSelectedDelegate(string text);
public event RadioButtonSelectedDelegate RadioButtonSelected;
public Form2()
{
InitializeComponent();
}
private void radioButton_CheckedChanged(object sender, EventArgs e)
{
RadioButton radioButton = (RadioButton)sender;
if (radioButton.Checked)
{
// 发出委托事件
RadioButtonSelected?.Invoke(radioButton.Text);
}
}
}
```
请注意,在Form1中我们使用了多线程来打开Form2,这是因为我们需要保持Form1的UI响应,同时在新线程中运行Form2。在Form2中选择RadioButton时,会触发Form1中的委托事件,并将选中的RadioButton的Text作为参数传递给Form1。然后,我们使用Invoke方法在UI线程上更新Form1中的txtbox控件。
这样,当点击Form1中的Button1时,Form2会打开并显示所选择的RadioButton的Text在Form1中的txtbox控件上。
阅读全文
相关推荐
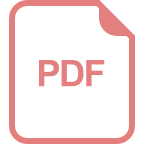
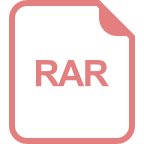
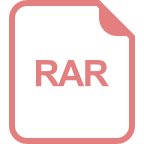
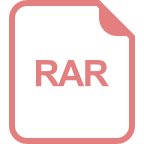
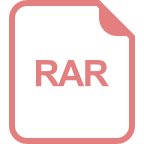
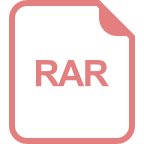
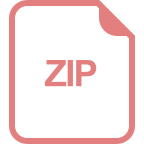
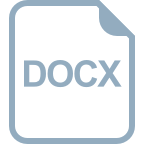
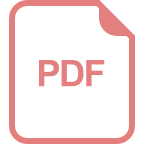
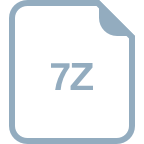
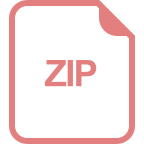
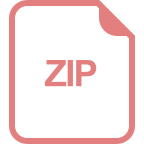
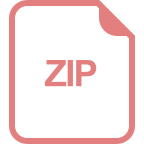
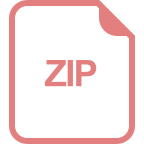
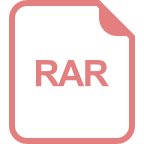
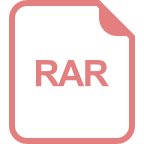