用c语言表示幂的末三位数
时间: 2024-12-02 11:21:41 浏览: 17
在 C 语言中,如果你想表示一个数字的幂运算的末三位数,可以使用取模运算 `%` 结合循环来实现。假设你想计算 `base` 的 `exponent` 次方的末三位,你可以这样做:
```c
#include <stdio.h>
#include <string.h>
// Function to calculate the last three digits of a power
char* last_three_digits(int base, int exponent) {
char result[4] = {'0', '0', '0', '\0'};
while (exponent > 0) {
if (exponent % 100 >= 10) { // If there are more than one digit in the current position
int temp = (base * (exponent % 10)) % 1000; // Calculate the next digit by multiplying and taking mod 1000
result[0] = temp % 10 + '0'; // Store the first digit
result[1] = (temp / 10) % 10 + '0'; // Store the second digit
result[2] = temp / 100; // Store the third digit as an integer, no need for modulus here
} else { // If it's just one or zero digits, multiply once and store directly
int temp = base * (exponent % 10);
result[0] = temp % 10 + '0';
exponent -= 10;
}
exponent /= 10; // Reduce the exponent for the next iteration
}
return result;
}
int main() {
int base, exponent;
printf("Enter base and exponent: ");
scanf("%d%d", &base, &exponent);
char* result = last_three_digits(base, exponent);
printf("Last three digits of %d^%d are: %s\n", base, exponent, result);
free(result); // Don't forget to free the dynamically allocated memory
return 0;
}
```
这个函数首先初始化一个字符数组 `result` 来存储结果,并通过循环逐步计算并更新每个位置的值,直到 `exponent` 变为零。
阅读全文
相关推荐
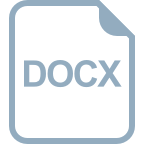
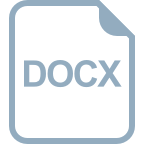
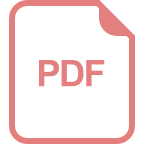
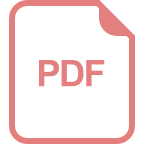
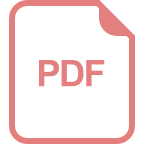
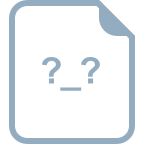
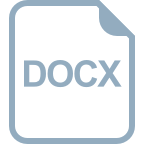
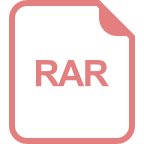
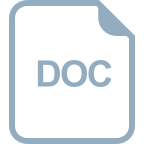
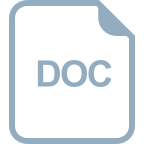
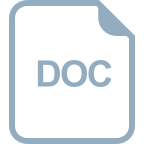
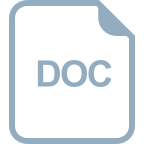
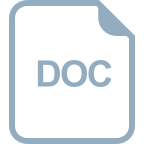
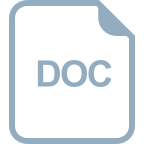