c++函数题计算向量内积
时间: 2024-09-26 07:05:44 浏览: 36
在C++中,计算两个向量的内积通常涉及到对应元素相乘然后求和的操作。内积也称为点积,它是一个标量值,等于两个向量x和y的每个分量相乘后的结果之和,数学上表示为 \( \vec{x} \cdot \vec{y} = x_1y_1 + x_2y_2 + ... + x_ny_n \)。
下面是一个简单的C++函数示例,用于计算两个一维向量(vector<int>)的内积:
```cpp
#include <iostream>
#include <vector>
int dotProduct(const std::vector<int>& vec1, const std::vector<int>& vec2) {
if (vec1.size() != vec2.size()) {
throw std::invalid_argument("Vectors must have the same size");
}
int result = 0;
for (size_t i = 0; i < vec1.size(); ++i) {
result += vec1[i] * vec2[i];
}
return result;
}
int main() {
std::vector<int> vecA = {1, 2, 3};
std::vector<int> vecB = {4, 5, 6};
try {
int innerProduct = dotProduct(vecA, vecB);
std::cout << "The dot product of vectors A and B is: " << innerProduct << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,`dotProduct` 函数接收两个`std::vector<int>`类型的参数,如果它们的长度不同则抛出异常。接着遍历这两个向量,将每个元素相乘并累加到结果变量中。
阅读全文
相关推荐
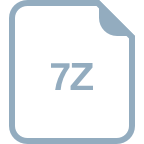
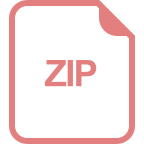
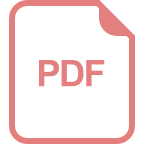
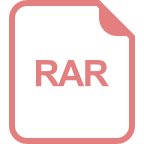
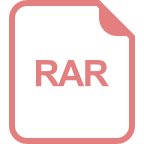
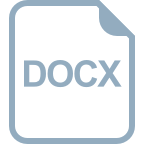
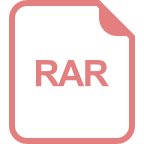
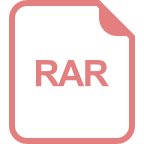
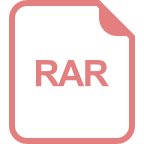
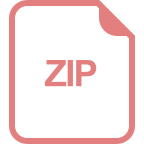
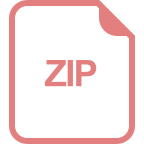
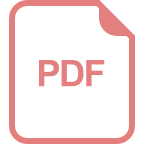
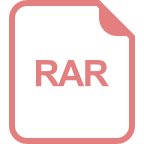
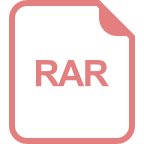
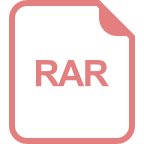
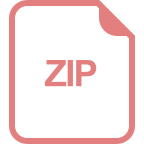
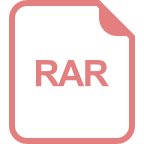
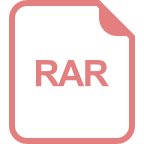
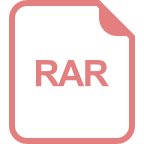