c语言多组字符串 删除指定字符
时间: 2023-12-08 20:03:41 浏览: 74
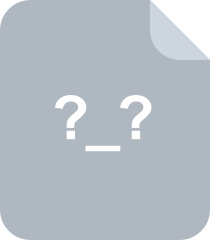
C语言程序设计-从字符串s中删除指定的字符c.c

以下是C语言中删除字符串中某个字符的程序的具体操作步骤:
1. 定义一个函数delete_string,该函数接收两个参数:一个字符数组str和一个字符ch。
2. 使用for循环遍历字符串str中的每个字符,如果当前字符不等于待删除字符ch,则将该字符添加到一个新的字符数组new_str中。
3. 将new_str中的内容复制回原来的字符数组str中,即可完成删除操作。
4. 在主函数中,从键盘输入一个字符串及一个待删除字符,调用delete_string函数进行删除操作,并输出删除后的字符串str。
以下是C语言中删除多组字符串中某个字符的程序的具体操作步骤:
1. 定义一个函数delete_char,该函数接收两个参数:一个字符数组str和一个字符ch。
2. 使用for循环遍历字符串str中的每个字符,如果当前字符不等于待删除字符ch,则将该字符添加到一个新的字符数组new_str中。
3. 将new_str中的内容复制回原来的字符数组str中,即可完成删除操作。
4. 在主函数中,定义一个二维字符数组strs,存储多组字符串,从键盘输入待删除字符ch,使用for循环遍历每个字符串,调用delete_char函数进行删除操作,并输出删除后的字符串。
```c
#include <stdio.h>
#include <string.h>
void delete_string(char str[], char ch) {
int len = strlen(str);
char new_str[len];
int j = 0;
for (int i = 0; i < len; i++) {
if (str[i] != ch) {
new_str[j++] = str[i];
}
}
new_str[j] = '\0';
strcpy(str, new_str);
}
void delete_char(char strs[][100], char ch) {
int n = sizeof(strs) / sizeof(strs[0]);
for (int i = 0; i < n; i++) {
delete_string(strs[i], ch);
printf("%s\n", strs[i]);
}
}
int main() {
char str[100];
char ch;
printf("请输入一个字符串:");
scanf("%s", str);
printf("请输入待删除字符:");
scanf(" %c", &ch);
delete_string(str, ch);
printf("删除后的字符串为:%s\n", str);
char strs[][100] = {"hello", "world", "happy", "birthday"};
printf("请输入待删除字符:");
scanf(" %c", &ch);
delete_char(strs, ch);
return 0;
}
```
阅读全文
相关推荐
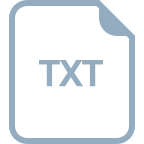
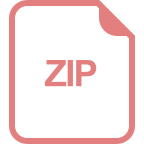
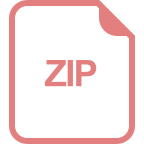
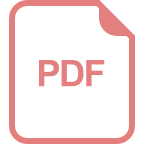
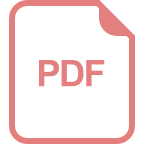
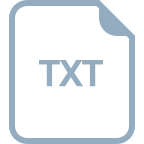
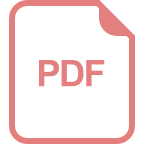
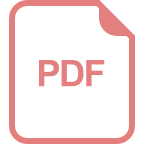