传入一个CString路径,得到路径的最后一个斜杠右边文件名
时间: 2024-09-09 21:05:38 浏览: 64
在C++中,如果你有一个CString类型的路径,要提取路径的最后一个斜杠右边的文件名(不包括斜杠),你可以使用`std::filesystem::path`类,它提供了一种方便的方式来解析和操作文件路径。这里是一个示例:
```cpp
#include <string>
#include <filesystem>
std::string lastPathComponent(const std::wstring& path) {
// 使用std::filesystem::path来解析路径
std::filesystem::path p(path);
// 获取最后一个分量,这将给我们文件名
return p.filename();
}
// 示例
std::wstring filePath = L"c:\\windows\\temp\\readme.txt";
std::string fileName = lastPathComponent(filePath);
```
在这个例子中,`lastPathComponent`函数会返回`"readme.txt"`。
相关问题
c++标准14,传入一个CString路径,得到路径的最后一个斜杠右边文件名
在C++14中,要从一个`CString`类型的路径中提取最后一个斜杠右边的文件名(不包括斜杠),你可以使用`std::filesystem`库,它是C++17引入的标准库的一部分,但在一些编译器中可能有对C++14兼容的实现。这里是一个示例:
```cpp
#include <filesystem>
#include <string>
std::string getLastFileName(const std::wstring& path) {
// 使用std::filesystem::path解析路径
std::filesystem::path p(path);
// 获取路径的最后部分,即文件名
return p.stem(); // .stem()返回不带扩展名的基础名称
}
// 转换为C++14兼容版本,如果需要的话
std::string getLastFileNameC14(const std::string& path) {
if (std::filesystem::exists(path)) { // 检查路径是否存在
std::wstring_convert<std::codecvt_utf8<wchar_t>> converter;
std::wstring wpath = converter.from_bytes(path); // 转换为宽字符
return getLastFileName(wpath);
}
else {
throw std::runtime_error("Path does not exist.");
}
}
```
这段代码首先使用`std::filesystem::path`解析输入路径,然后通过`.stem()`获取文件名。注意,对于C++14版本,你需要手动转换`CString`到`std::string`,因为`std::filesystem`不直接支持`CString`。
c++标准14,传入一个CString路径,得到路径的最后一个斜杠右边文件名,不使用filesystem
在C++14中,你可以通过手动解析字符串来达到这个目的。由于C++的标准库并不直接提供获取路径文件名的功能,但可以利用`std::string`的方法和成员函数。以下是一个简单的示例:
```cpp
#include <string>
std::string getFileNameWithoutPath(const std::string& path) {
size_t lastSlashPos = path.rfind("\\"); // 查找最后一个斜杠的位置(对于Windows)
if (lastSlashPos != std::string::npos) { // 如果找到
return path.substr(lastSlashPos + 1); // 返回从该位置到字符串结束的部分
} else { // 如果未找到,可能只有一个反斜杠(Linux/Unix)
lastSlashPos = path.rfind("/");
return path.substr(lastSlashPos + 1);
}
}
// 使用示例
std::string fullPath = "C:\\Users\\example\\Documents\\myFile.txt";
std::string fileName = getFileNameWithoutPath(fullPath);
```
请注意,这个方法假设路径是以斜杠(/ 或 \)结尾的。如果路径可能以其他字符结尾,你可能需要添加额外的逻辑来处理这种情况。
阅读全文
相关推荐





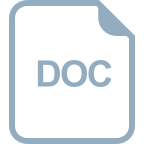
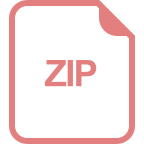








