java springboot 线程池 创建线程池 任务执行完毕关闭线程池
时间: 2024-08-23 11:00:25 浏览: 39
在Java Spring Boot中,创建线程池是一个常见的任务管理机制,它可以帮助我们高效地管理和复用线程资源。Spring Boot提供了`ThreadPoolTaskExecutor`这个工具类来简化线程池的配置和管理。
以下是创建线程池的基本步骤:
1. 配置:首先,在Spring的配置文件`application.properties`或`application.yml`中添加线程池的相关设置,例如线程数、队列大小、线程名称等:
```yaml
spring:
task:
executor:
pool-size: 5 # 线程池大小
queue-capacity: 10 # 线程队列容量
thread-name-prefix: "MyTask-" # 线程名称前缀
```
2. 创建bean:然后在Spring配置中定义一个`ThreadPoolTaskExecutor` bean,并注入到需要使用的bean中:
```java
import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor;
@Configuration
public class ThreadPoolConfig {
@Bean(name = "threadPoolTaskExecutor")
public ThreadPoolTaskExecutor threadPoolExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10); // 最大并发数
executor.setQueueCapacity(10); // 定义工作队列大小
executor.setThreadNamePrefix("my-task-executor-");
return executor;
}
}
```
3. 使用线程池:现在你可以通过@Autowired注解获取这个线程池实例,并提交Runnable或Callable任务:
```java
@Service
public class MyService {
private final ThreadPoolTaskExecutor executor;
@Autowired
public MyService(ThreadPoolTaskExecutor executor) {
this.executor = executor;
}
public void executeTask(Runnable task) {
executor.execute(task);
}
// 或者使用Future
public Future<?> submitCallable(Callable<?> callable) {
return executor.submit(callable);
}
}
```
4. 关闭线程池:通常情况下,Spring Boot会在应用退出时自动关闭线程池。但在某些场景下,如果需要手动关闭,可以在适当的地方调用`shutdown()`方法,如`@PreDestroy`方法:
```java
@Override
@PreDestroy
public void destroy() {
executor.shutdown(); // 调用此方法停止接收新的任务
try {
if (!executor.awaitTermination(60, TimeUnit.SECONDS)) { // 等待60秒让所有任务完成
executor.shutdownNow(); // 强制结束剩余任务
}
} catch (InterruptedException e) {
executor.shutdownNow(); // 取消中断并立即结束任务
Thread.currentThread().interrupt();
}
}
```
相关推荐
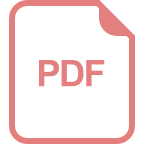
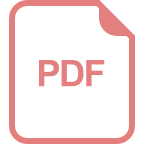
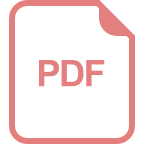















