Напиши игру тетрис на python
时间: 2024-04-30 21:16:49 浏览: 149
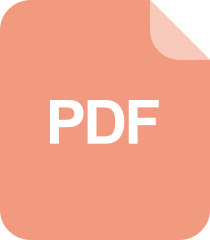
使用Python写一个小游戏
当然,我可以为您提供一个简单的示例来编写Python的俄罗斯方块游戏(Tetris)。以下是一个基本的实现示例:
```python
import pygame
import random
# 游戏窗口大小
WINDOW_WIDTH = 800
WINDOW_HEIGHT = 600
# 方块大小
BLOCK_SIZE = 30
# 方块颜色
COLORS = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128, 128)
]
# 方块形状
SHAPES = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 1], [1, 0, 0]],
[[1, 1, 1], [0, 0, 1]]
]
def draw_block(surface, x, y, color):
pygame.draw.rect(surface, color, (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
def draw_board(surface, board):
for y in range(len(board)):
for x in range(len(board[y])):
draw_block(surface, x, y, COLORS[board[y][x]])
def check_collision(board, shape, x, y):
for row in range(len(shape)):
for col in range(len(shape[row])):
if shape[row][col] and (x + col >= len(board) or x + col < 0 or y + row >= len(board) or board[y + row][x + col]):
return True
return False
def rotate_shape(shape):
return list(zip(*reversed(shape)))
def clear_rows(board):
full_rows = [row for row in range(len(board)) if all(board[row])]
for row in full_rows:
del board[row]
board.insert(0, * len(board))
return len(full_rows)
def main():
pygame.init()
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("Tetris")
clock = pygame.time.Clock()
board = [ * (WINDOW_WIDTH // BLOCK_SIZE) for _ in range(WINDOW_HEIGHT // BLOCK_SIZE)]
current_shape = random.choice(SHAPES)
current_x = WINDOW_WIDTH // BLOCK_SIZE // 2 - len(current_shape) // 2
current_y = 0
score = 0
game_over = False
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if not check_collision(board, current_shape, current_x - 1, current_y):
current_x -= 1
elif event.key == pygame.K_RIGHT:
if not check_collision(board, current_shape, current_x + 1, current_y):
current_x += 1
elif event.key == pygame.K_DOWN:
if not check_collision(board, current_shape, current_x, current_y + 1):
current_y += 1
elif event.key == pygame.K_UP:
rotated_shape = rotate_shape(current_shape)
if not check_collision(board, rotated_shape, current_x, current_y):
current_shape = rotated_shape
if not check_collision(board, current_shape, current_x, current_y + 1):
current_y += 1
else:
for row in range(len(current_shape)):
for col in range(len(current_shape[row])):
if current_shape[row][col]:
board[current_y + row][current_x + col] = len(COLORS) - 1
score += clear_rows(board)
current_shape = random.choice(SHAPES)
current_x = WINDOW_WIDTH // BLOCK_SIZE // 2 - len(current_shape) // 2
current_y = 0
if check_collision(board, current_shape, current_x, current_y):
game_over = True
screen.fill((255, 255, 255))
draw_board(screen, board)
for row in range(len(current_shape)):
for col in range(len(current_shape[row])):
if current_shape[row][col]:
draw_block(screen, current_x + col, current_y + row, COLORS[len(COLORS) - 1])
pygame.display.flip()
clock.tick(5)
pygame.quit()
if __name__ == "__main__":
main()
```
这是一个简单的俄罗斯方块游戏的实现,使用了Pygame库来进行图形界面的绘制和交互。您可以在Python环境中运行此代码,即可开始游戏。
阅读全文
相关推荐
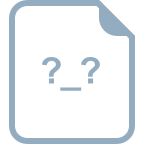


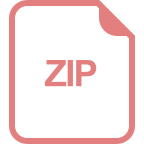
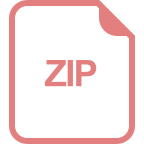
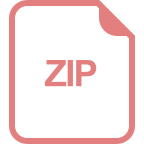
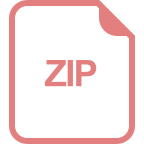
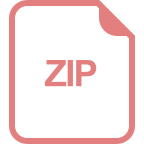
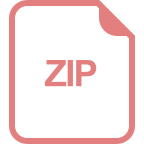
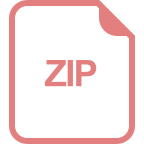





